Displaying images in Matlab figures has always been sort-of awkward. We need to read the image file into a Matlab matrix (possibly with accompanying colormap), then create an invisible axis in our figure window, and finally display the image data within this axis, typically with the imagesc function. In practice, this is not as difficult as it may seem, and can be done in 3 lines of code (imread(); axes(); imagesc();). To display images in uicontrols, we need to set their CData property with the image data. Unfortunately, this is only supported for static (non-animated) images.
But what if we want to display an animated image? There are several image formats that enable animation, GIF being the most well-known. Animated GIFs are used ubiquitously, for anything from logos to simple visualizations. I often use them myself in my utilities and this blog, to illustrate functionality [1] or usage [2]. Creating animated GIFs is so easy — I often use Picasion [3], but there are numerous alternatives.
So let’s assume that I have the animated GIF featured here:
This example serves as the logo in a demo live trading application [4] that I’ll present tomorrow at the MATLAB Computational Finance Conference [5].
To display this image in a Matlab GUI, we could use a mini-browser control that we can easily integrate [6] within the figure. But an even simpler and less resource-intensive solution is to integrate an HTML panel [7]:
hFig = figure('Color','w');
je = javax.swing.JEditorPane('text/html', '
');
[hj, hc] = javacomponent(je,[],hFig);
set(hc, 'pos', [10,10,70,70])
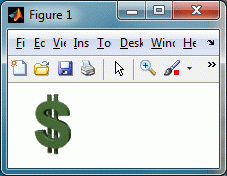
Notes:
- Although the GIF image is 64×64 pixels, we set the containing
JEditorPane
‘s size to 70×70, to account for the border and a small margin. You can obviously play with the size. - The image source was specified using the
file:/
protocol, as I explained here [8].
If our animated GIF uses transparency, we should set the container’s background to the same color as the figure, as I explained here [9]:
bgcolor = num2cell(get(hFig,'Color'));
hj.setBackground(java.awt.Color(bgcolor{:}));
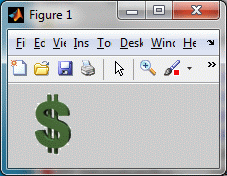
For anyone wondering, we could just as easily have used a simple
JLabel
rather than a JEditorPane
(see here [10]). The only difference is that we would need to also take care of the background:
hFig = figure('Color','w');
je = javax.swing.JLabel('
');
je.setBackground(java.awt.Color(1,1,1)); %=white
[hj, hc] = javacomponent(je,[],hFig);
set(hc, 'pos', [10,10,70,70])
We can similarly display animated GIFs on any uicontrol, due to the inherent nature of uicontrols that they accept HTML contents [11]. So, we can place our animated GIF in buttons, drop-downs, listboxes etc. It’s not as straight-forward in drop-downs/listboxes as it is in buttons, but then again not many people need animated images in drop-downs or listboxes as they do in buttons. For push/toggle buttons, checkboxes and radio-buttons, displaying animated GIFs is trivially simple:
h1 = uicontrol('style','push', 'pos', [10,10,70,70], 'String','
');
h2 = uicontrol('style','check', 'pos', [90,10,70,70], 'String','
');
h3 = uicontrol('style','radio', 'pos',[170,10,70,70], 'String','
');
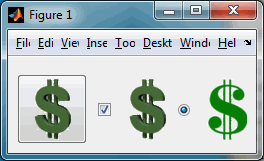
The standard documented manner of displaying animated GIFs in Matlab is to imread all image frames from the GIF file, then start a timer that will periodically replace the axes image (or uicontrol CData property) with the next image frame in an endless loop. This is relatively tedious to set up, results in noticeable flicker and is relatively CPU intensive. I believe that the undocumented mechanism I’ve explained above is simpler to code and lighter on graphic resources.
Today’s article was a tribute to two old friends, whom I never met in person so far: a client who has recently asked me about this issue, and a member of a Graphics team who said he liked my related posts. I hope to meet both of them at the conference tomorrow. If you are interested in any aspect of computational finance, you will surely find some interesting presentations at this conference. So if you’re in town, I urge you to attend (free registration [12]; my presentation is scheduled for 4:50pm). Otherwise, we could meet anywhere in New York tomorrow (Friday May 24), to discuss how I could bring value to your needs, either financial-oriented or not.
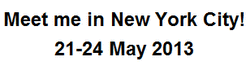
16 Comments To "Displaying animated GIFs"
#1 Pingback By Real-time trading system demo | Undocumented Matlab On May 29, 2013 @ 06:15
[…] Undocumented Matlab Charting Matlab’s unsupported hidden underbelly Skip to content HomeAboutConsultingTrainingIB-MatlabMatlab-Java bookTODOPoliciesSite map ← Displaying animated GIFs […]
#2 Pingback By Rich-contents log panel | Undocumented Matlab On September 19, 2013 @ 09:36
[…] Animated GIF logo image (rotating Dollar sign) […]
#3 Pingback By Animated busy (spinning) icon | Undocumented Matlab On April 16, 2014 @ 11:02
[…] We can also simply embed the animated GIF image directly in our GUI, as I explained here. […]
#4 Comment By derphelix On July 13, 2014 @ 14:29
Thank you very much for charing this undocumented feature with us.
But i wonder: Do you know how i could delete the the javax.swing.JEditorPane ?
Everytime I’m trying to delte the hc variable, matlab will use more memory than before the deletion (seen in the TaskManager).
#5 Comment By Yair Altman On July 13, 2014 @ 16:07
Clearing the Matlab variable that holds the Java object’s reference should enable the Java object to be garbage-collected by the JVM.
#6 Pingback By Transparency in uicontrols | Undocumented Matlab On December 10, 2014 @ 11:02
[…] note: I have shown another alternative for displaying clickable transparent images in my article on displaying animated/transparent GIFs. Readers might also find interest in the related article on transparent uipanels. Next week I plan […]
#7 Comment By lalitkumar annaldas On February 15, 2015 @ 06:17
hey,
but what if i wanted to display the animated gif file on one of the axes present in the GUI of MATLAB instead of displaying it on external figure window.
If you can help me please forward the code to my mail ID:-
[19]
#8 Comment By Yair Altman On February 15, 2015 @ 06:30
@Lalitkumar – if you insist on using an axes (why???), then you could use a timer to refresh the displayed image frame at a certain frequency.
#9 Comment By Greg Mason On March 17, 2015 @ 07:45
This post has been very helpful, but now I’m trying to use the deploytool to package my GUI for distribution. Unfortunately when it’s packaged the address still maps back to my local drive and the GIF doesn’t work – is there a way to hide / embed the GIF in the package so the end user won’t see the picture file in their directory but still have it show up in the GUI?
Thanks again.
#10 Comment By Yair Altman On March 17, 2015 @ 08:51
@Grep – you could place the GIF on some website (either your own or some public image-sharing site) and let the HTML img src point to the relevant URL rather than to local disk file. Of course, the user would need to have internet connection to that URL in order to see the GIF.
#11 Comment By Greg Mason On March 17, 2015 @ 10:55
Unfortunately one of the reasons for distributing is when people are not connected to the inter/intranet they can still run the programs without needing a matlab license.
However, I appear to have found a workaround. The below code is in my .m file and the file “Pic_For_Error.gif” is included in the Shared Resources and Helper Files section of deploytool (the same section that the .fig file is included). Hope this helps anyone that may want to distribute their GUIs with GIFs!
#12 Comment By Yair Altman On March 17, 2015 @ 10:59
@Greg – you said you didn’t want the image to appear in the deployment folder, but that’s exactly what you did above…
#13 Comment By Greg Mason On March 17, 2015 @ 11:32
Sorry – maybe my vernacular was not correct. What I did not want was for the GIF file to be seen by the end user. They would only see the .exe, that way the GIF will always work whether or not they move the .exe between folders and they wouldn’t be able to change the location/delete the GIF either.
When I use the deploytool as described with the code above, the end result is a just a single .exe file that can be moved and distributed yet still has a functioning GIF. The end user will only see the .exe which was my goal.
#14 Comment By Yair Altman On March 18, 2015 @ 14:47
@Greg – I assume that you are aware of the fact that the compiled binary is simply a self-extracting exe that anyone can open using winzip and see the internal image resources (although not the code – those files are encrypted).
#15 Comment By ME On March 30, 2015 @ 09:36
thank you very much !!
What if I want to hide a GIF that was already displayed ?
#16 Comment By ME On March 30, 2015 @ 10:06
… using a pushbutton