I would like to introduce guest blogger Scott Koch. Scott is part of the development team at Eigenvector Research Inc. [1], makers of PLS_Toolbox [2], a commercial chemometrics and multivariate data analysis toolbox. Today Scott will expand on JGraph, a Java-based open source graph visualization and diagramming library.
What is JGraph?
JGraph is an interactive Java-based diagramming and graph visualization library. The maintainers of JGraph have a product family that includes a Java Swing library (open-source), a JavaScript library (proprietary commercial), as well as other technologies. They call their product family “mxGraph”, and the Swing product specifically as “JGraphX”. The Swing library is available on GitHub [3] and licensed under BSD. I will refer to the Swing library as JGraph, since that was the original library’s name until it was completely redesigned some years ago and renamed JGraphX. You can read additional information on JGraph and access various documentation alternatives in its README file [3].
Graphing is a powerful visualization tool that has not yet been integrated into the core Matlab product. I assume that this will be rectified by MathWorks in a future Matlab release. But until that time, or even later if you are still using R2015a or older, we do not have a solution in the core Matlab. In our toolbox product, we use a cleverly designed image to display a simple diagram, but I always thought it would be nice to have a “real” diagramming tool that was interactive and full-featured.
So, as a long time follower of Yair’s blog, I was thrilled to see his post [4] several years ago about integrating Java libraries for charting and diagramming in Matlab figures. Unfortunately, my first attempts at getting JGraph to work in Matlab were not very successful. Thanks to a comment [5] on that original post by Markus Behle, I was able to gain full access to JGraph in Matlab. Today’s post will show how we can use JGraph in Matlab.
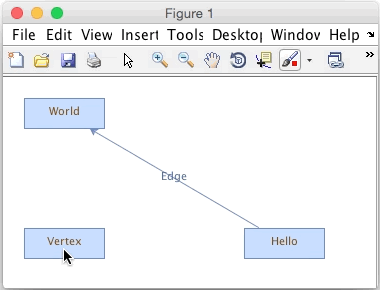
Creating a simple graph
To install JGraph, first download [6] the JGraph zip file, extract it in some folder, and then add the contained JGraph jar file to your Matlab’s Java path:
javaaddpath('jgraphx/lib/jgraphx.jar');
Having installed JGraph, we can now create graphs pretty easily in Matlab – see Yair’s original post [4] for details.
Unfortunately, dragging doesn’t work and that’s a bummer. To enable dragging, create a custom graph component. I posted a followup comment [7] showing how to do this. Note that the following code will work without creating a custom graph component but you won’t be able to drag (and drop).
In JGraph, a graph is made up of cells. A cell can be a vertex (node) or edge (connector). A cell can also hold other cells in a group.
Below is a code snippet similar to Yair’s original JGraph post, except we’ll use the custom graph component from above and add an additional vertex:
% Make the graph object
graph = javaObjectEDT('mymxGraph’);
% If not using custom graph component use the following
% graph = javaObjectEDT('com.mxgraph.view.mxGraph');
% Get the parent cell
parent = graph.getDefaultParent();
% Group update
graph.getModel().beginUpdate();
% Add some child cells
v1 = graph.insertVertex(parent, '', 'Hello', 240, 150, 80, 30);
v2 = graph.insertVertex(parent, '', 'World', 20, 20, 80, 30);
v3 = graph.insertVertex(parent, '', 'Vertex', 20, 150, 80, 30);
graph.insertEdge(parent, '', 'Edge', v1, v2);
graph.getModel().endUpdate();
% Get scrollpane
graphComponent = com.mxgraph.swing.mxGraphComponent(graph);
% Make a figure and stick the component on it
f = figure('units','pixels');
pos = get(f,'position');
mypanel = javax.swing.JPanel(java.awt.BorderLayout);
mypanel.add(graphComponent);
[obj, hcontainer] = javacomponent(mypanel, [0,0,pos(3:4)], f);
% Normalize units so resize works better
set(hcontainer,'Units','normalized');
We have 3 vertices and a single edge connecting two vertices. Each has a label. By default you can edit the labels and drag the vertices and edges. If you mouse over the middle of a vertex it will highlight, indicating that if you click and drag, you will be able to add an edge. Dragging the edge to another vertex will connect it. You can also resize and reposition vertices, as well as set edge labels, as shown in the animated image above.
Customizing graphs with HTML and CSS
If you look at online examples of JGraph, you can see there are lots of options for customizing your graph’s appearance. JGraph uses “styles” on vertices and edges, which are similar in concept to Cascading Style Sheets (CSS). You can add style information when you create a vertex, or by using the Graph Model. Rerun the code above with the following lines substituted:
v1 = graph.insertVertex(parent, '', 'Hello', 240, 150, 150, 150, 'rounded=1;');
v2 = graph.insertVertex(parent, '', 'World', 20, 20, 80, 30, 'rounded=1;strokeColor=blue;fillColor=lightblue');
v3 = graph.insertVertex(parent, '', 'Vertex', 20, 150, 80, 30, 'rounded=1;strokeColor=#000000;fillColor=#00CCFF;gradientColor=#333300;fontColor=white');
graph.insertEdge(parent, '', 'Edge', v1, v2, 'edgeStyle=elbowEdgeStyle;strokeColor=#5d65df;strokeWidth=2');
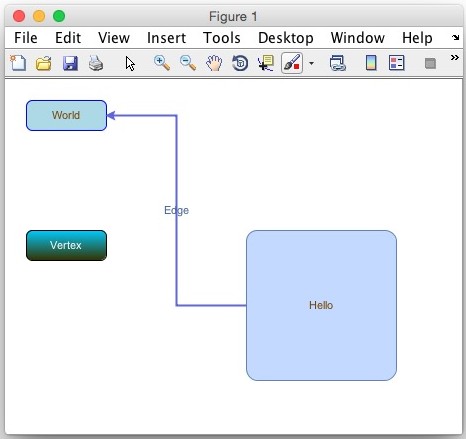
As Yair has taught us, most Java controls can render HTML. JGraph is no different, you just need to turn it on and add HTML to your label:
>> graph.setHtmlLabels(true);
>> htmltxt = ['My HTML Vertex
'...
'
Style | Value |
rounded | 1 |
fillColor | yellow |
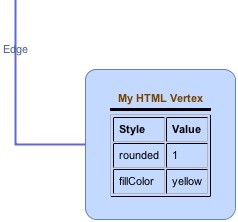
Adding a callback
Let’s add more functionality to our graph by adding a callback. To do this, we need to add a mouse-clicked callback to the graph scrollpane:
% Handle the scrollpane and add a click callback
scrollpanehandle = handle(graphComponent.getGraphControl, 'CallbackProperties');
set(scrollpanehandle, 'MouseClickedCallback', {@mouseClickCallback,f})
With a click callback in place, we can interrogate the click event and graph to determine what object is under the click. If the click happens over a vertex, a context menu is displayed allowing changing of the fill color.
function mouseClickCallback(Obj,eventData,myFig)
%Graph mouse click callback
%Extract graph object
graphcomponent = Obj.getGraphContainer;
graph = graphcomponent.getGraph;
figpos = get(myFig,'position');
%Get cell under click if it's there
mycell = graphcomponent.getCellAt(eventData.getX,eventData.getY);
if isempty(mycell) || mycell.isEdge
%Clicking in open space
return
end
if javax.swing.SwingUtilities.isRightMouseButton(eventData)
mymenu = findobj(myFig,'tag','backgroundmenu');
if isempty(mymenu)
%Create context menu
mymenu = uicontextmenu('tag','backgroundmenu');
uimenu(mymenu,'Tag','graymenu','Label','Gray Background');
uimenu(mymenu,'Tag','bluemenu','Label','Blue Background');
end
%Update callback with current cell object
set(findobj(mymenu,'tag','graymenu'),'callback',{@changeFillColor,graph,mycell,'gray'});
set(findobj(mymenu,'tag','bluemenu'),'callback',{@changeFillColor,graph,mycell,'blue'});
%Show context menu
set(mymenu,'position', [eventData.getX+8 figpos(4)-eventData.getY-8]);
set(mymenu,'visible','on');
end
end
function changeFillColor(Obj,eventData,varargin)
% Change cell fill color
%Pull graph object, current cell object, and new color value out of varargin
mygraph = varargin{1};
mycell = varargin{2};
newcolor = varargin{3};
%Get current style
mystyle = char(mycell.getStyle);
%Chop string into cells and locate fillcolor cell
stylecell = strsplit(mystyle,';');
fcloc = ~cellfun('isempty',strfind(stylecell,'fillColor='));
%Make new fillcolor string
switch newcolor
case 'gray'
newcolor = 'fillColor=#E8E8E8';
case 'blue'
newcolor = 'fillColor=#A9BCF5';
end
%Add new color into style
if any(fcloc)
stylecell{fcloc} = newcolor;
else
stylecell{end+1} = newcolor;
end
%Join styles back into single style string
mystyle = strjoin(stylecell,';');
%Set new style
mygraph.getModel.setStyle(mycell,mystyle);
end
Conclusions
Hopefully this post gave enough information to get started making attractive and useful graphs with Matlab and JGraph. There is a lot of functionality included with JGraph and we’ve only scratched the surface today. I hope to have a future post discussing Groups, Drag and Drop, Layout Engines, and a few other topics that build upon what was discussed today.
Here are a few resources that could help you get started:
- Online (JavaScript) demo: https://www.draw.io [8]
- Style demo: http://blog.draw.io/mxgraph-cell-styles [9]
- JGraph Forum: http://forum.jgraph.com/questions [10]
- List of styles (properties starting with “
STYLE_
“): https://github.com/jgraph/jgraphx/blob/master/src/com/mxgraph/util/mxConstants.java [11] - Yair’s post on integrating JGraph and JGraphT in Matlab: https://undocumentedmatlab.com/articles/jgraph-and-bde [4]
Addendum September 4, 2015: Matlab R2015b has just been released and includes very interesting new functionality supporting graph algorithms and visualization (additional details [12]).
8 Comments To "JGraph in Matlab figures"
#1 Comment By Sam Roberts On July 2, 2015 @ 05:14
Hi Scott and Yair,
I’ve used JGraphX quite a bit within MATLAB UIs, and have a couple of suggestions based on my experience.
i) When you create the mxGraphComponent, I’d make sure to explicitly put it on the EDT, as you’ve done in your post for the mxGraph. In other words, rather than
I’d write
That should make sure there are no threading issues.
ii) Rather than making a JPanel, adding the graphcomponent to the JPanel, and then using javacomponent to insert the JPanel into a figure as you do above, I would just make a regular MATLAB uipanel in the figure, and then use javacomponent to place the graphcomponent into the uipanel. A uipanel is basically a JPanel anyway, but again it has wrappers that will manage any threading issues that might arise.
iii) I’ve found that, by default, the graphcomponent contains several mouse listeners that interfere with callbacks that I’m trying to create. So I’ve found it useful to remove them all before creating my own. You can do this using something like the following code:
iv) Instead of
javax.swing.SwingUtilities.isRightMouseButton(eventData)
, you can just useeventData.getButton
, which equals 1 for the left button or 3 for the right button. You can also useeventData.isShiftDown
oreventData.isControlDown
to detect key modifiers on the mouse click.Hope that’s useful for people!
Sam
#2 Comment By Sam Roberts On July 2, 2015 @ 05:17
PS – I don’t know if you’ve downloaded it yet, but the prerelease of R2015b does actually contain a new suite of functionality for graphs. There’s a new graph object, and it has lots of great functionality for graph-theoretic work, such as shortest paths and minimum spanning trees. It also contains graph layout and plotting functions – unfortunately, at the moment these are not even 1% as nice looking as JGraph. But I’m sure they’ll be improving that in future releases.
#3 Comment By Yair Altman On July 2, 2015 @ 05:35
@Sam – thanks for the detailed feedback 🙂
#4 Comment By Vivek Shankar On April 12, 2017 @ 22:43
Hello,
Thanks for the excellent tutorial. After spending some time with the JgraphX api, I am able to accomplish most of the things I need. One thing I am unable to get my head around is the saving and loading of a JGraph objects in Matlab. I had tried to use the encode and decode codecs in the JgraphX library and then to write as an XML output, but for some reason the codecs dint seem to work =- encoding the graph-model results in an empty variable. I was trying :
#5 Comment By mukesh Muthu On March 9, 2021 @ 21:02
i get the following error , im using matlab 2016b-64bit
if i use
if i use
i checked the dynamic javapath and it has the mentioned jar file listed
#6 Comment By Yair Altman On March 9, 2021 @ 21:18
Perhaps you tried to access the class before you used
javaaddpath
to add the JAR file to the classpath. If Matlab fails to find a class, then it will not be able to access it even after adding to the classpath – the only solution is to restart Matlab and to remember to add the JAR file to the classpath before trying to access the class.#7 Comment By mrv On May 9, 2022 @ 07:00
hello,
I used MATLAB 2019b update9, I have add jgraphx.jar to javaclassapth, and restart matlab, but still got errors below:
#8 Comment By Yair Altman On May 9, 2022 @ 11:39
The version of JGraph that you downloaded uses a newer version of Java (11) than the one that Matlab supports (8). You need to either (1) find an earlier version of JGraph that uses Java 8 (or earlier), or alternatively (2) make Matlab use Java 11 (or later) run-time engine (JRE) . Among these two alternatives, #1 is safer and easier.