A few days ago, one of my consulting clients asked me to help him with a very strange problem: he had a Matlab class having a constant
property that holds a reference to some handle class object. The problem was that when he tried to modify the property’s inner values he got Matlab run-time errors because the inner value apparently remained unmodified!
Here is a distilled version of my client’s classes:
classdef (Abstract) MainClass
properties (Constant)
inner = InnerClass
end
methods (Static)
function setInnerValue(newValue)
MainClass.inner.value1 = newValue;
end
end
end
classdef InnerClass < handle
properties
value1
value2
end
end
And the strange run-time behavior:
>> MainClass.inner.value1 = 5 MainClass = inner: [1x1 struct] >> MainClass.inner.value2 % causes a strange run-time error! Reference to non-existent field 'value2'. >> MainClass.inner.value1 % strange - value1 appears unmodified! ans = [] >> MainClass.inner % strange - value1 appears ok here, but where is value2 ?! ans = value1: 5 >> MainClass.setInnerValue(7) % another strange run-time error! Reference to non-existent field 'setInnerValue'. >> clear classes % let's try it afresh... >> MainClass.setInnerValue(7) % looks ok, no error... >> MainClass.inner % strange - now we have both value1 & value2, but value1 is not updated! ans = InnerClass with properties: value1: [] value2: [] >> MainClass.inner.value1 = 9 % one last attempt, that also fails! MainClass = inner: [1x1 struct] >> MainClass.inner ans = InnerClass with properties: value1: [] value2: [] >> MainClass.inner.value1 ans = []
Understanding the [buggy?] behavior
What the heck is going on here? did Matlab's MCOS flip its lid somehow? Well, apparently not. It turns out that all these strange behaviors can be attributed to a single Matlab oddity (I call it a "bug") in its class object system (MCOS) implementation. Understanding this oddity/bug then leads to a very simply workaround.
The underlying problem is that Matlab does not understand MainClass.inner.value1
to mean "the value1
property of the inner
property of the MainClass
class". Matlab does understand MainClass.inner
to mean "the inner
property of the MainClass
class", but adding another dereferencing level (.value1
) in the same Matlab expression is too complex for MCOS, and it does not properly understand it.
This being the case, Matlab's internal interpreter reverts to the simplistic understanding that MainClass.inner.value1
means "the value1
field of the struct inner
, which is itself a field of the outer struct named MainClass
". In fact, this creates a new struct variable named MainClass
in our current workspace. Still, due to Matlab's internal precedence rules, the MainClass
class overshadows the new variable MainClass
, and so when we try to read (as opposed to update) MainClass.inner
we are actually referencing the inner reference handle of the MainClass
class, rather than the corresponding field in the new struct. The reference handle's value1
property remains unmodified because whenever we try to set MainClass.inner.value1
to any value, we're just updating the struct variable in the local workspace.
Unfortunately, no run-time warning is issued to alert us of this. However, if we load MainClass.m in the Matlab editor, we get a somewhat-cryptic MLint warning that hints at this:
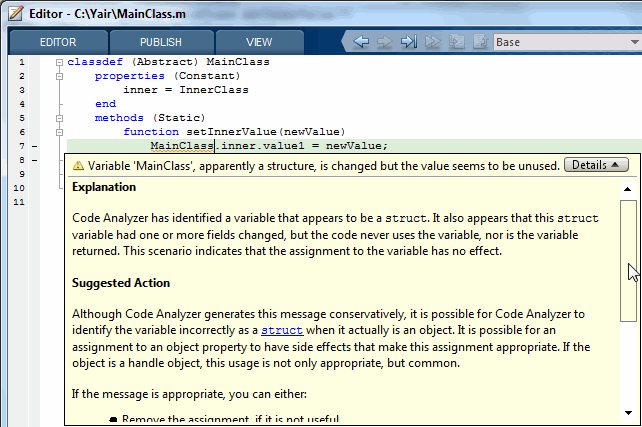
Confusing? indeed!
(However, refer to the reader comments below, which provide insight into the reasoning of what's going on here, and contend that this is actually the expected behavior. I will let the readers decide for themselves whether they agree or not.)
The workaround
Now that we understand the source of all the problems above, the solution is easy: help Matlab's internal interpreter understand that we want to reference a property of the inner object reference. We do this by simply splitting the 3-element construct MainClass.inner.value1
into two parts, first storing innerObj = MainClass.inner
in a temporary local variable (innerObj
), then using it to access the internal innerObj.value1
property:
innerObj = MainClass.inner;
innerObj.value1 = 7;
Hopefully, in some future Matlab release, MCOS will be smarter than today and automatically handle multi-element MCOS constructs, as well as it currently does for 2-element ones (or multi-element Java/.Net/COM constructs).
Have you ever encountered any other Matlab bug that appears perplexing at first but has a very simple workaround as above? If so, then please share your findings in a comment below.
10 Comments To "Solving a Matlab MCOS bug"
#1 Comment By Sven On December 2, 2015 @ 12:24
There was something like this bug in R2015a, which seems to have been fixed in R2015b.
In order to access the elements of a cell-type variable, you would first need to store a copy of that variable locally.
The above now works in 2015b. In 2015a however, the result was:
Whereas you could work around with:
#2 Comment By Sven On December 2, 2015 @ 12:25
And to clarify: the above bug was limited to “cell-typed variables of *tables*”.
#3 Comment By Dave Foti On December 2, 2015 @ 14:12
I can understand Yair’s confusion, but there is a simple explanation the behavior described in this post (with Constant properties). MATLAB doesn’t have variable declarations. When a name appears on the left of an equal sign, that statement always create a variable from the left-most name. Try “MainClass.inner = 1” and you will see that it doesn’t matter how complex the indexing expression is, assignment always introduces a new variable that hides any function or class of the same name. In this case the dot indexing means that MainClass will be a struct. It is important that variables have highest precedence so that scripts and functions don’t have to worry about collisions between the variable names used in the code and functions and classes on the path (a set of names that is very large and always growing).
Reference to a name that has not been introduced as a variable will find the class. It doesn’t matter how many dots you have or how complex the indexing expression is. Here are some functions you can try that show how MATLAB consistently treats assignment as creating a variable. Note that the last two functions produce the same results whether or not MainClass exists on the path.
This comes down to the fact that preserving the meaning of variables independent of what is on the path is considered more important than making it easy to create mutable per-class state (which is often problematic anyway because it can lead to unwanted cross-talk between applications that share the same libraries).
#4 Comment By Yair Altman On December 2, 2015 @ 15:21
@Dave – thanks for your clarification, but the inconsistency here between the assignment and reference precedence rules is confusing. I contend that the following precedence rule might be more intuitive and consitent in such cases, while still preserving the default behavior for the remainder of the cases:
1. try to understand MainClass (in both assignment and referencing) as referring to variable MainClass on the local workspace. If successful then continue to use this variable.
2. otherwise, try to understand MainClass as a class/package name. If successful and no error, then continue to use this. Note that this does not break your requirement for MainClass=5 to override the class definition and create a local variable named MainClass, because assigning to a class is illegal and therefore raises an error and falls-through to the following rule:
3. otherwise, in an assignment create a new workspace variable named MainClass, and in a reference raise an error about trying to use an unrecognized identifier.
These precedence rules will ensure that variables always take precedence over function/class/package names (as you have rightfully pointed out), but only when they exist – otherwise the existing function/class/package name should obviously take precedence over the non-existing variable. The behavior now becomes fully consistent across assignment and reference, and I think is much more intuitive in run-time than the current situation.
#5 Comment By Dave Foti On December 3, 2015 @ 07:22
Hi Yair,
The problem is that it isn’t enough for “MainClass = 1” to ensure that MainClass is a variable. As long as structs have existed in MATLAB, it has been possible to create structs using the syntax “MainClass.inner = 1” just as numeric arrays can be created using indexing as in “X(1:10) = 1” Thus, your proposed rules would still mean that a function or script (like the 2 createStruct examples I provided) could be written using local struct variables that later got re-interpreted as referring to a class and its property that happened to be on the path.
#6 Comment By Brad Stiritz On December 2, 2015 @ 21:02
Hi Yair, thanks for a super-interesting post. Thanks to Dave as well for the helpful clarification. Just to clarify your workaround, Yair, here’s a revised implementation of the static function, and the expected result
produced by the new code:
#7 Comment By Yair Altman On December 3, 2015 @ 00:16
@Brad – indeed so 🙂
#8 Comment By alexandre On December 4, 2015 @ 04:10
cool post!
I am confused about the following point:
The inner class is declared inside the ‘constant’ properties – why would we be assigning to it in the first place? It seems to me that this is key for a problem to occur (there is no other attribute that I can see that could be accessed via the static function call – [7])
Reading the docs above suggeststhat Constant properties can be modified (but not by sub-classes). But this article suggests that they should be set at definition and not touched thereafter: [8].
So are matlab (Constant) properties what other OO languages call ‘static’ properties?
#9 Comment By Yair Altman On December 4, 2015 @ 04:16
The property is constant in the sense that its reference handle remains unmodified after being set, but its inner properties are not constant. It’s like saying that a company’s ID remains constant, but its employees, phone number, and physical address may possibly change over time. Or like saying that a house address remains constant but its occupants change over time.
Related post: [9]
#10 Comment By Jeroen Boschma On January 7, 2016 @ 04:35
Hi Yair,
I think this is certainly not a simple MCOS parser bug, even not a bug at all, but since I have limited knowledge about the inner things of MATLAB classes I may be on thin ice here…
Try the following as your very first statement:
So that rules out that there is only a simple MCOS parser bug just because there would be too many dereferencing levels. Since there is no variable MainClass, MATLAB correctly dereferences all levels within the class MainClass.
The underlying problem, I think, is that you cannot change class definitions, only instances of classes. What in this case happens is the following:
1) Within ‘setInnerValue()’ there is no variable named MainClass, and MATLAB detects the reference to the class, correctly taking all reference levels into account (my example above shows that this is not the problem).
2) MATLAB detects the assignment statement.
3) MATLAB falls back on the rule “modifying class definitions (i.e. its properties) is not possible”.
4) MATLAB falls back on the only remaining option: create a new struct variable ‘MainClass’ and execute the assignment.
So I think the bottom line is that MATLAB has a strict interpretation of “modifying class definitions is not possible”, and that therefore every direct assignment via the class definition is prohibited. This is not a bug at all in my opinion, but you end up in a discussion how tight the underlying fields of ‘inner’ are related to MainClass itself. The fact that you can change the fields of ‘inner’ using a workaround does not mean that MATLAB should allow doing this directly via the class definition itself. For this matter, I tend to agree with MATLAB’s choice.