I was recently asked by a client to add a few buttons labeled “1”-“4” to a GUI toolbar. I thought: How hard could that be? Simply get the toolbar’s handle from the figure [1], then use the builtin uipushtool function to add a new button, specifying the label in the String property, right?
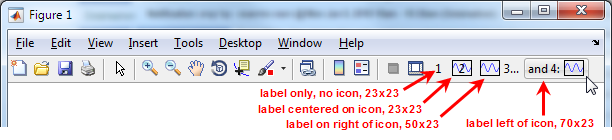
Well, not so fast it seems:
hToolbar = findall(hFig, 'tag','FigureToolBar'); % get the figure's toolbar handle uipushtool(hToolbar, 'String','1'); % add a pushbutton to the toolbar Error using uipushtool There is no String property on the PushTool class.
Apparently, for some unknown reason, standard Matlab only enables us to set the icon (CData) of a toolbar control, but not a text label.
Once again, Java to the rescue:
We first get the Java toolbar reference handle, then add the button in standard Matlab (using uipushtool, uitoggletool and their kin). We can now access the Java toolbar’s last component, relying on the fact that Matlab always adds new buttons at the end of the toolbar. Note that we need to use a short drawnow to ensure that the toolbar is fully re-rendered, otherwise we’d get an invalid Java handle. Finally, once we have this reference handle to the underlying Java button component, we can set and customize its label text and appearance (font face, border, size, alignment etc.):
hToolbar = findall(hFig, 'tag','FigureToolBar'); % get the figure's toolbar handle
jToolbar = hToolbar.JavaContainer.getComponentPeer; % get the toolbar's Java handle
for buttonIdx = 1 : 4
% First create the toolbar button using standard Matlab code
label = num2str(buttonIdx); % create a string label
uipushtool(hToolbar, 'ClickedCallback',{@myCallback,analysisIdx}, 'TooltipString',['Run analysis #' label]);
% Get the Java reference handle to the newly-created button
drawnow; pause(0.01); % allow the GUI time to re-render the toolbar
jButton = jToolbar.getComponent(jToolbar.getComponentCount-1);
% Set the button's label
jButton.setText(label)
end
The standard Matlab toolbar button size (23×23 pixels) is too small to display more than a few characters. To display a longer label, we need to widen the button:
% Make the button wider than the standard 23 pixels
newSize = java.awt.Dimension(50, jButton.getHeight);
jButton.setMaximumSize(newSize)
jButton.setPreferredSize(newSize)
jButton.setSize(newSize)
Using a text label does not prevent us from also displaying an icon: In addition to the text label, we can also display a standard icon (by setting the button’s CData property in standard Matlab). This icon will be displayed to the left of the text label. You can widen the button, as shown in the code snippet above, to make space for both the icon and the label. If you want to move the label to a different location relative to the icon, simply modify the Java component’s HorizontalTextPosition property:
jButton.setHorizontalTextPosition(jButton.RIGHT); % label right of icon (=default)
jButton.setHorizontalTextPosition(jButton.CENTER); % label on top of icon
jButton.setHorizontalTextPosition(jButton.LEFT); % label left of icon
In summary, here’s the code snippet that generated the screenshot above:
% Get the Matlab & Java handles to the figure's toolbar
hToolbar = findall(hFig, 'tag','FigureToolBar'); % get the figure's toolbar handle
jToolbar = hToolbar.JavaContainer.getComponentPeer; % get the toolbar's Java handle
% Button #1: label only, no icon, 23x23 pixels
h1 = uipushtool(hToolbar);
drawnow; pause(0.01);
jButton = jToolbar.getComponent(jToolbar.getComponentCount-1);
jButton.setText('1')
% Create the icon CData from an icon file
graphIcon = fullfile(matlabroot,'/toolbox/matlab/icons/plotpicker-plot.gif');
[graphImg,map] = imread(graphIcon);
map(map(:,1)+map(:,2)+map(:,3)==3) = NaN; % Convert white pixels => transparent background
cdata = ind2rgb(graphImg,map);
% Button #2: label centered on top of icon, 23x23 pixels
h2 = uipushtool(hToolbar, 'CData',cdata);
drawnow; pause(0.01);
jButton = jToolbar.getComponent(jToolbar.getComponentCount-1);
jButton.setText('2')
jButton.setHorizontalTextPosition(jButton.CENTER)
% Button #3: label on right of icon, 50x23 pixels
h3 = uipushtool(hToolbar, 'CData',cdata);
drawnow; pause(0.01);
jButton = jToolbar.getComponent(jToolbar.getComponentCount-1);
jButton.setText('3...')
d = java.awt.Dimension(50, jButton.getHeight);
jButton.setMaximumSize(d); jButton.setPreferredSize(d); jButton.setSize(d)
% Button #4: label on left of icon, 70x23 pixels
h4 = uipushtool(hToolbar, 'CData',cdata);
drawnow; pause(0.01);
jButton = jToolbar.getComponent(jToolbar.getComponentCount-1);
jButton.setText('and 4:')
jButton.setHorizontalTextPosition(jButton.LEFT)
d = java.awt.Dimension(70, jButton.getHeight);
jButton.setMaximumSize(d); jButton.setPreferredSize(d); jButton.setSize(d)
Many additional toolbar customizations can be found here [2] and in my book “Undocumented Secrets of MATLAB-Java Programming [3]“. If you’d like me to design a professional-looking GUI for you, please contact me [4].
Caveat emptor: all this only works with the regular Java-based GUI figures, not web-based (“App-Designer”) uifigures.