Since Matlab 7.0 (R14), Matlab has included a built-in GUI table control (uitable), at first as a semi-documented [1] function and in release 7.6 (R2008a) as a fully-documented [2] function. Useful as this control is, it lacks many features that are expected in modern GUIs, including sorting, filtering, cell-specific appearance and behavior, gridline customization etc. In past articles [3] I have explained how uitable can be customized to achieve a more professional-looking table. I expanded on this in my book [4] and my detailed uitable customization report [5].
Today I explain how a grouping hierarchy can be achieved in a table control that can be used in Matlab GUI. Such a control, which is a combination of a uitree [6] and uitable, is typically called a tree-table. We can find numerous examples of it in everyday usage. I have encapsulated the functionality in a utility called treeTable on the Matlab File Exchange (#42946 [7]). The utility is provided with full source code and open-source license, and readers are welcome to use and modify it. A detailed explanation of the technicalities follows below, but if you’re just interested in having a sortable, rearrangeable, customizable, groupable table control, then all you need to do is download and use the utility.
headers = {'ID','Label','Logical1','Logical2','Selector','Numeric'};
data = {1,'M11',true, false,'One', 1011; ...
1,'M12',true, true, 'Two', 12; ...
1,'M13',false,false,'Many',13.4; ...
2,'M21',true, false,'One', 21; ...
2,'M22',true, true, 'Two', 22; ...
3,'M31',true, true, 'Many',31; ...
3,'M32',false,true, 'One', -32; ...
3,'M33',false,false,'Two', 33; ...
3,'M34',true, true, 'Many',34; ...
};
selector = {'One','Two','Many'};
colTypes = {'label','label','char','logical',selector,'double'};
colEditable = {true, true, true, true, true}
icons = {fullfile(matlabroot,'/toolbox/matlab/icons/greenarrowicon.gif'), ...
fullfile(matlabroot,'/toolbox/matlab/icons/file_open.png'), ...
fullfile(matlabroot,'/toolbox/matlab/icons/foldericon.gif'), ...
}
% Create the table in the current figure
jtable = treeTable('Container',gcf, 'Headers',headers, 'Data',data, ...
'ColumnTypes',colTypes, 'ColumnEditable',colEditable, ...
'IconFilenames',icons, 'Groupable',true, 'InteractiveGrouping',false);
% Collapse Row #6, sort descending column #5 (both are 0-based)
jtable.expandRow(5,false); % 5=row #6; false=collapse
jtable.sortColumn(4,true,false); % 4=column #5; true=primary; false=descending
The basic implementation concept
Unfortunately, uitable as-is cannot be customized to have groupable rows. It derives from JIDE’s SortableTable
, rather than one of its groupable derived classes. On the other hand, uitree (don’t you agree that after a decade of this so-useful function being semi-documented it ought to be made official?) uses a different class hierarchy outside com.jidesoft.grid
, and so it cannot be easily customized to display rows (as opposed to simple labels).
These limitations mean that we cannot use uitable or uitree and need to use a custom component. Luckily, such a component is available in all Matlab installations, on all platforms. It is part of the grid components package, on which Matlab GUI has relied for many years, by JIDE Software [8]. JIDE Grids is a collection of components that extend the standard Java Swing JTable [9] component, and is included in each Matlab installation (/java/jarext/jide/jide-grids.jar under the Matlab root). I discussed multiple JIDE controls [10] in this blog over the years. You can find further details on JIDE Grids in the Developer Guide [11] and the Javadoc documentation [12].
In fact, there are no less than three different components that we could use in our case: TreeTable
, GroupTable
and HierarchicalTable
:
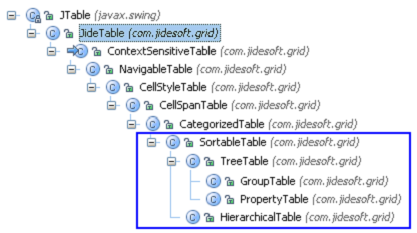
Note that we cannot use
PropertyTable
since that component is limited to only two columns. This is perfect for presenting property names and values, which is the reason it is used by Matlab’s inspect function and my generic propertiesGUI utility [13] or Levente Hunyadi’s Property Grid utility [14]. But in this case we wish to display a general multi-column table, so PropertyTable
is a no-go.TreeTable
and GroupTable
enable data rows that have similar type (class), whereas HierarchicalTable
enables more flexibility, by allowing display of any component type (including full tables) in child rows. This flexibility comes with a price that customizing a HierarchicalTable
is more difficult than TreeTable
or GroupTable
. These latter two components are quite similar; we use GroupTable
, which extends TreeTable
with the ability to automatically group rows that have the same value in a certain column.
Data model
The above-mentioned table classes all derive from SortableTable
(which also underlies uitable). Unfortunately, something in the Matlab-Java interface breaks the ability of the JIDE table classes (or rather, their data model) to understand numeric values for what they are. As a result, sorting columns is done lexically, i.e. “123” < “34” < “9”. To fix this, I’ve included a custom Java table model (MultiClassTableModel
) with the treeTable utility, which automatically infers the column type (class) based on the value in the top row (by overriding the getColumnClass() method).
Using this new class is pretty easy:
% Create the basic data-type-aware model using our data and headers
javaaddpath(fileparts(mfilename('fullpath'))); % add the Java class file to the dynamic java class-path
model = MultiClassTableModel(data, headers); % data=2D cell or numeric array; headers=cell array of strings
% Wrap the standard model in a JIDE GroupTableModel
com.mathworks.mwswing.MJUtilities.initJIDE; % initialize JIDE
model = com.jidesoft.grid.DefaultGroupTableModel(model);
model.addGroupColumn(0); % make the first column the grouping column
model.groupAndRefresh; % update the model data
% Use the generated model as the data-model of a JIDE GroupTable
jtable = eval('com.jidesoft.grid.GroupTable(model);'); % prevent JIDE alert by run-time (not load-time) evaluation
jtable = handle(javaObjectEDT(jtable), 'CallbackProperties'); % ensure that we're using EDT
% Enable multi-column sorting
jtable.setSortable(true);
% Present the tree-table within a scrollable viewport on-screen
scroll = javaObjectEDT(JScrollPane(jtable));
[jhscroll,hContainer] = javacomponent(scroll, tablePosition, hParent);
set(hContainer,'units', 'normalized','pos',[0,0,1,1]); % this will resize the table whenever its container is resized
Here, com.mathworks.mwswing.MJUtilities.initJIDE is called to initialize JIDE’s usage within Matlab. Without this call, we may see a JIDE warning message in some Matlab releases. We only need to initJIDE once per Matlab session, although there is no harm in repeated calls.
javacomponent is the undocumented built-in Matlab function that adds Java Swing components to a Matlab figure, using the given dimensions and parent handle. I discussed it here [15].
Callbacks
There are two main callbacks that can be used with treeTable:
- table data update – this can be set by uncommenting line #237 of treeTable.m:
set(handle(getOriginalModel(jtable),'CallbackProperties'), 'TableChangedCallback', {@tableChangedCallback, jtable});
which activates the sample
tableChangedCallback()
function (lines #684-694). Naturally, you can also set your own custom callback function.% Sample table-editing callback function tableChangedCallback(hModel,hEvent,jtable) % Get the modification data modifiedRow = get(hEvent,'FirstRow'); modifiedCol = get(hEvent,'Column'); label = hModel.getValueAt(modifiedRow,1); newData = hModel.getValueAt(modifiedRow,modifiedCol); % Now do something useful with this info fprintf('Modified cell %d,%d (%s) to: %s\n', modifiedRow+1, modifiedCol+1, char(label), num2str(newData)); end % tableChangedCallback
- row selection update – this is currently enabled in line #238 of treeTable.m:
set(handle(jtable.getSelectionModel,'CallbackProperties'), 'ValueChangedCallback', {@selectionCallback, jtable});
which activates the sample
selectionCallback()
function (lines #696-705). Naturally, you can also set your own custom callback function.% Sample table-selection callback function selectionCallback(hSelectionModel,hEvent,jtable) % Get the selection data MinSelectionIndex = get(hSelectionModel,'MinSelectionIndex'); MaxSelectionIndex = get(hSelectionModel,'MaxSelectionIndex'); LeadSelectionIndex = get(hSelectionModel,'LeadSelectionIndex'); % Now do something useful with this info fprintf('Selected rows #%d-%d\n', MinSelectionIndex+1, MaxSelectionIndex+1); end % selectionCallback
Some useful features of treeTable
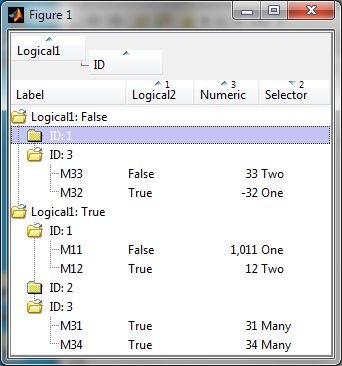
- Sortable columns (including numeric columns)
- Rearrangeable columns (drag headers left/right)
- Auto-fit the table columns into the specified container width
- Manually resize columns by dragging the column divider gridlines (not just the header dividers as in uitable)
- Flat or groupable table, based on the specified Groupable parameter (default=true)
- Ability to interactively group columns (just like Microsoft Outlook) using the InteractiveGrouping parameter (default=false)
- Selector cells only show the drop-down arrow of the currently-selected cell (unlike uitable which shows it for all the column cells)
- Selector cells enable editing, unlike uitable that only enables selecting among pre-defined values
- Ability to attach user-defined icons for the leaf rows and the grouping rows (expanded/collapsed)
- Easily attach custom cell editors or renderers to any table column (see my book [4] and my detailed uitable customization report [5] for details)
All of these features can be turned on/off using the control’s properties. Again, see my book or report for details (or ask me [16] to do it for you…).
I remind readers that I will be visiting clients in Austria (Vienna, Salzburg) and Switzerland (Zurich) in August 18-29. If you live in the area, I will be happy to meet you to discuss how I could bring value to your needs, as consultant, contractor or trainer (more details [17]).
90 Comments To "treeTable"
#1 Comment By Jim Hokanson On August 9, 2013 @ 20:04
Wow, this looks perfect for a couple of projects that I have in mind. I look forward to looking at the code and trying it out. Thanks.
#2 Comment By Assaf On August 12, 2013 @ 04:16
Quick Q here..
Is there a way to do an “automatically” subgrouping — i.e. Directly set it in the source code (and not in the interactive way)?
thx.
#3 Comment By Yair Altman On August 12, 2013 @ 04:20
@Assaf – It’s good to see you here!
You can do something similar to
#4 Comment By Assaf On August 12, 2013 @ 04:34
Hi, Yair.
Thanks for the quick reply!
For some reason your suggestion didn’t work for me\
This is what I got:
———-
>> model.addGroupColumn(1);
No appropriate method, property, or field addGroupColumn for class com.jidesoft.grid.SortableTreeTableModel.
———–
#5 Comment By Yair Altman On August 12, 2013 @ 04:37
I had a typo in the code snippet – it’s now fixed
#6 Comment By Assaf On August 12, 2013 @ 04:43
thanks!!
#7 Comment By Assaf On September 3, 2013 @ 02:23
Another Quick Q here..
How can I update the “selector” (the drop-down list) on the fly — i.e. I want to add \ remove entries on the list – based on values entered into other cells.
Many Thanks!
Assaf
#8 Comment By Alex On September 10, 2013 @ 01:20
I have noticed that
returns a different value depending on how the TreeTable rows are sorted. Is there a way to tell the corresponding row of the data cell array when a row in the TreeTable has been selected?
#9 Comment By Yair Altman On September 11, 2013 @ 15:58
This will get you the original data model row number (note: 0-based value):
#10 Pingback By Using JIDE combo-boxes | Undocumented Matlab On October 16, 2013 @ 06:11
[…] into this wealth of components in our own GUI. I’ve recently shown an example of this in my treeTable utility, which greatly extends Matlab’s standard uitable. After using treeTable for a while, […]
#11 Comment By Sam On October 17, 2013 @ 06:37
Hi Yair,
I’d like to adjust the tableChanged callback in the function I’m writing (perform a plotting task when a box is checked/unchecked) and leave treeTable.m generic. I’m only familiar with the basic set(handles.xxx,’Callback’,{@updateplot}) syntax and I’m not sure how to adjust for this type of object, could you point me in the right direction? I’ve been playing around with the first block of code on this page as an example.
Thanks!
-Sam
#12 Comment By kefang On December 18, 2013 @ 00:26
hi Yair
i’d like to use a timer to update the data in treetable.how can i realize it ?
Thaks!
frank
#13 Comment By Yair Altman On December 18, 2013 @ 05:17
Matlab timers are described [24]
#14 Comment By kefang On December 18, 2013 @ 18:16
hi Yair
i know matlab timers. my question is how to change the data in treetable when i use matlab timers callback function?
Thanks! could you leave me your email?
frank
#15 Comment By Yair Altman On December 18, 2013 @ 18:32
@Kefang – You can use the setValueAt method. To understand how to use it, refer to any Java table tutorial.
Re my email, there’s a link on the top-right of every page on this blog. For personal assistance via email I usually charge a consulting fee.
#16 Comment By kefang On December 18, 2013 @ 19:14
thank you for your help.
#17 Comment By Viktor On January 10, 2014 @ 18:53
Hi Yair,
many thanks for another fine hack!
I have a questions regarding the treeTable:
So far i have not been able to remove the treeTable from a figure. I’ve managed to hide it
by using the setVisible(false) method but couldn’t use the space for anything else afterwards.
So is it possible to remove the treeTable from the container i linked it to?
Cheers,
Viktor
#18 Comment By Yair Altman On January 11, 2014 @ 09:26
@Viktor – right now there is no easy way to remove the treeTable. You can delete the Matlab container
hcontainer
from lines 200-204 if you store it somewhere (e.g., the treeTable’s ApplicationData property using setappdata/getappdata).If you don’t have the
hcontainer
handle, you can directly remove it from view as follows:#19 Comment By Kevin On January 21, 2014 @ 09:18
This is awesome. I would like to have a hierarchical tree. I got some sample data to create a uitree, and understand adding uinodes to the uitree are connected by:
To get:
After some tinkering with the sample data for treetable, I was unable to get a hierarchy (sub-folders grouped under sub-folders), although the construct of the data is similar to the uinode. I am confused if I am just formatting the sample data wrong, or if what I am hoping to achieve relies on a deeper issue being addressed:
Also, I would like to know how to add data to the displayed folder rows. Currently they are empty, but I would like to add numeric values to the leaf nodes, and have parent branches contain roll-up values for the child leaves (and other child sub-branches) beneath it.
Thanks,
-Kevin
#20 Comment By Kevin On January 22, 2014 @ 06:46
Sorry – in my above post I meant to say ‘I would like to have a hierarchical tree with a table to the right of it’. So, I want a treetable functionality where I can put data and widgets, and yet have the full hierarchy for data in the first column.
#21 Comment By JY On April 12, 2023 @ 22:20
Yair, could you respond to this question please? I’m also finding it difficult to implement such a multi-hierarchy table. Thanks
#22 Comment By Yair Altman On April 12, 2023 @ 23:24
I posted my
treeTable
utility 10 years ago for anyone to use freely, on an as-is basis, without any warranty, updates or support. If you need any customization or assistance with it, please email me (altmany at gmail) for private consulting.#23 Comment By kefang On February 9, 2014 @ 20:34
hi,
how can i get the row and col in the treetable when i use the function selectionCallback
#24 Comment By Yair Altman On February 13, 2014 @ 04:29
#25 Comment By frankie On February 17, 2014 @ 00:43
hi
i want to set different color in the odd and even row.how can i do it ?
#26 Comment By Yair Altman On February 17, 2014 @ 01:56
@Frankie – you will need to use a custom table cell renderer for this, as described [25] or in my [26].
#27 Comment By Jan On February 19, 2014 @ 10:31
Hi again,
I’m having a problem with the treeTable utility. As soon as I call the treeTable function, my workspace gets cleared. I haven’t seen a ‘clear xxx;’ or anything in your code. Can you help me?
Thanks again,
Jan
#28 Comment By Yair Altman On February 19, 2014 @ 10:48
@Jan – [27]… This undocumented side-effect of javaaddpath has been reported numerous years ago, and was never fixed.
#29 Comment By Jan On February 20, 2014 @ 09:53
Hi Yair,
then I have to deal with this somehow …
Another thing: why is the ‘ValueChangedCallback’ called twice?
I apologize for those many questions I had for the last few days…
Thanks,
Jan
#30 Comment By Yair Altman On February 20, 2014 @ 10:00
@Jan – I’m sorry but if you would like dedicated support, I would be happy to provide it for you as a consultant. Please approach me via email (link at the top-right of this page).
#31 Comment By frankie On February 20, 2014 @ 20:07
Hi Yair
In the following codes ,
groupStyle.setBackground(java.awt.Color(.1,.2,.3));
No appropriate method, property, or field getGroup for class com.jidesoft.grid.DefaultGroupTableModel.
model.getGroup.setBackground(java.awt.Color(.1,.2,.3));
#32 Comment By Yair Altman On February 21, 2014 @ 03:27
@Frankie – there are variations between JIDE releases used by different Matlab releases. This specific method probably does not exist on your specific Matlab release. You’d need to search for alternatives, it is possible that in your particular release the method is called something else, or perhaps the functionality can be done by some other means. If you’d like me to investigate your specific case as a consultant, then email me.
#33 Comment By James On March 13, 2014 @ 10:02
Very nice. Is there a way to programmatically select or de-select a row? When I try
I get no appropriate method, and when I try
I get something like ‘changing ‘SelectedRows’ property of …. is not allowed. I also tried it with ‘SelectedRow’, but that gives the same errors.
Thank you for all of help!
Best regards,
#34 Comment By Yair Altman On March 13, 2014 @ 10:53
@James – use the changeSelection(rowIdx,colIdx,flag,flag) to programmatically set the selected row(s). More details in my book and uitable customization report.
#35 Comment By Geert On May 4, 2014 @ 07:15
HI Yair,
Is it easy to add additional columns to an existing tree table?
Geert
#36 Comment By Yair Altman On May 4, 2014 @ 14:33
@Geert – look at the sample usage. The columns are added/defined dynamically, based on the supplied data.
#37 Comment By Geert van Kempen On May 6, 2014 @ 09:03
Hi Yair,
Thanks for your reply, but I meant whether there is an way in which an user could simply add an (empty) new column interactively?
#38 Comment By Yair Altman On May 6, 2014 @ 09:12
not interactively – only programmatically. If you want interactivity, you can add uicontrols such as the one that I added in my [28].
#39 Comment By Julien On May 6, 2014 @ 12:47
Hello Yair,
Nice post !! Is it possible to display data in the cells of the rows which are expandable ?
#40 Comment By Yair Altman On May 6, 2014 @ 14:21
@Julien – take a look at the screenshots: the expandable columns automatically display their data in [columnName]: [value] format
Maybe I did not understand your question…
#41 Comment By Julien On May 7, 2014 @ 06:02
Actually, I would like to display statistics of the grouped rows at the expanded rows levels.
For example: I have a table which first column is a label (column on which grouping is done) and the other columns are Numbers. I would like to display on the expandable rows the mean values of all the expanded rows in the corresponding cells. First column will contain indeed the [columnName]: [value] and the others will contain the means (no spanning).
TableModel example :
After grouping on the first column :
Maybe it is clearer?
#42 Comment By Yair Altman On May 7, 2014 @ 10:28
You will probably need to use some Java class code for this, I don’t think it can be done directly in Matlab.
#43 Comment By LG On June 3, 2014 @ 07:20
Is there a simple way to add and control a uitree to a guide created gui ?
#44 Comment By Yair Altman On June 3, 2014 @ 08:11
@LG – you cannot (as of R2014a) do it from within the GUIDE application, but once you create your application with GUIDE you can modify the generated m-file to add the corresponding Matlab commands, for example in the *_OpeningFcn function at the top of the m-file.
#45 Comment By Geert van Kempen On June 19, 2014 @ 12:52
Hi Yair,
Is it possible to make a particular row editable instead of cloumns?
Thanks,
Geert
#46 Comment By Yair Altman On June 19, 2014 @ 13:26
I don’t think so…
#47 Comment By vinod chauhan On July 5, 2014 @ 03:45
sir can i get the full source code of treetable
#48 Comment By Yair Altman On July 5, 2014 @ 11:04
@Vinod – simply download it from the File Exchange link provided in the article – it includes the full source code
#49 Comment By Peter Dowell On July 26, 2014 @ 08:04
Hi Yair,
I’ve come accross an interesting issue running TreeTable.
When I sub group a column either interactively, or programatically as described above, columns which previously were non-editable become editable.
For example, if you call TreeTable as per the code in the article, except with:
Everything works fine (i.e. you cannot edit the columns), until you run:
At which point, the columns become editable again. Any suggestions?
#50 Comment By Yair Altman On July 26, 2014 @ 18:53
@Peter – indeed: when you add a grouping column you affect the model and the old definitions are forgotten. You can do the following (a variant can be found in the treeTable source code):
#51 Comment By Peter Dowell On July 28, 2014 @ 12:58
@Yair,
Thanks for the quick reply. I guess the states of the renderers/editors would have to be updated when the user changes the grouping. I was thinking that the setColumnRenderersEditors function could be called by a listener function on the re-grouping event. What do you think?
#52 Comment By Yair Altman On July 28, 2014 @ 13:03
Sounds reasonable. You’re welcome to try. Come back and post a followup comment here after you figure out the exact specifics.
#53 Comment By ss On May 25, 2017 @ 15:40
I can edit by a double-click even the column is non-editable . I don’t know what happened
#54 Comment By Ruedi On January 19, 2015 @ 10:11
Hi Yair,
Thanks for this great GUI table control. One more question: is there an easy way to include kind of a “total” row? For e.g. in your figure above, if the ID3 has some total (column Numeric may sum up to 1) I would like to show this sum in the same row as the ID3-icon.
Thanks for your help
#55 Comment By Yair Altman On January 31, 2015 @ 16:48
@Ruedi – there is no automated way to add a total row AFAIK, but you can always add it programmatically, and use some [29] to make it stand out from the data rows.
#56 Comment By Simon Spagnol On February 24, 2015 @ 19:06
In treeTable.m there are references to setTableData and getTableData. Where exactly are these functions defined?
Alternatively thought I could something like
jtable.setModel(javax.swing.table.DefaultTableModel(jData,jHeader));
jtable.repaint;
to update the treeTable panel.
#57 Comment By Yair Altman On February 25, 2015 @ 10:18
@Simon – setTableData() and getTableData() are simply placeholders for functions to get/set the data from the table. They are not defined anywhere, they are merely used to illustrate a sample row insertion function. You would need to implement these functions in your own code.
#58 Comment By Markus Jakobsen On March 10, 2015 @ 14:51
Hi Yair
Why do I get this error when I run the following code?
Undefined function ‘getTableData’ for input arguments of type
‘javahandle_withcallbacks.com.jidesoft.grid.GroupTable’
#59 Comment By Yair Altman On March 10, 2015 @ 16:09
@Markus – I answered this exact question [30]
It’s right there above your comment – didn’t you see it?
#60 Comment By Markus Jakobsen On March 11, 2015 @ 01:58
Hi Yair
I saw it, but must have misunderstood the meaning of “placeholders”. So the only way to get the entire data table is to run the following through a loop? Or?
How do I append data to an existing table? I can’t access rows beyond the data size with setValueAt.
So all in all, could you give a few pointers on how to get the entire dataset and set new data to an existing jtable.
Thanks a lot
Markus
#61 Comment By Yair Altman On March 12, 2015 @ 07:33
@Markus – you could do that, plus various other underlying Java methods to do this, which is beyond the scope of a blog comment. You can read about this in my [31] or my more recent/detailed [32]. Or [33]…
#62 Comment By Ruedi On March 11, 2015 @ 08:27
Hi Yair,
Trying the example above, the “numeric” column is not shown as doubles but as chars, i.e. left-aligned in the cell. Even with
Is there any additional java library I need to include in the code?
Thanks,
Ruedi
#63 Comment By Yair Altman On March 12, 2015 @ 07:40
@Ruedi – the treeTable.zip file includes various Java files – they should all be placed in the same folder as the treeTable.m Matlab file.
#64 Comment By Alex On March 18, 2015 @ 05:40
Hi Yair,
do you see any easy way to implement data filtering as it is done in Excel? (i.e., offer the user the possibility to restrict the display to the only rows containing a given user-selected value in a given column)
Cheers,
Alex
#65 Comment By Yair Altman On March 18, 2015 @ 14:48
@Alex – I explain how to do it (in two different manners) in my [32].
#66 Comment By Clayton Valentine On March 27, 2015 @ 06:37
Hi Yair,
I was wondering how to set the significant decimal digits for the table?
Thanks,
Clayton
#67 Comment By Marc On April 22, 2015 @ 07:51
Hi,
Thank you very much for this work. It is very handy.
I was wondering if we were any way to adjust ColumnWidth ?
Thanks,
Marc
#68 Comment By Alex On May 8, 2015 @ 05:57
Hi Yair,
to prevent editability of cells, I see in your code that you take two actions (in the subfunction setColumnRenderersEditors):
In practice, this, however, does not prevent the user to enter in some sort of in-between editable mode when starting to type in a cell after having selected it with the mouse; upon exiting the cell, the tableChangedCallback function is fired. The effect is equivalent to setting the number of clicks to start edition to zero but without having the cell content selected.
How could one prevent that from happening? That is, if a user starts typing, nothing happens at all and the callback function is not fired? Thanks for your reply.
Best,
Alex
#69 Comment By Yair Altman On May 8, 2015 @ 07:04
@Alex – have you actually tried this? I do not think that the cells can be interactively edited after editing has been prevented as in my code.
#70 Comment By Alex On May 8, 2015 @ 10:07
I have. I have sent you by e-mail a MWE showing this behavior.
#71 Comment By Alex On May 12, 2015 @ 01:18
A quick follow up on the issue mentioned above. Even though it’s a bit of a dirty fix—which kills some of the flexibility—it appears that blocking editing directly in the java class (MultiClassTableModel.java) by explicitly defining the isCellEditable method seems to prevent that unexpected behavior…
#72 Comment By Petr On May 25, 2016 @ 19:35
Works perfect, thanks Yair! Let me ask you how do I get the real row number in the table if my above label is collapsed? Thanks, Petr
#73 Comment By Thomas On December 21, 2016 @ 09:11
Hi Yair
I really like the flexibility your code offers. I have been toying with using your treetable to manage input data in a Matlab GUI for a while now, as this would offer a great improvement over what is available within documented Matlab. Only thing is that I cannot see how to retrieve the values of treetable components (e.g. true or false for checkboxes) beyond the row selection callback example in treetable.m. Normally I would set the ‘tag’ property when calling uicontrol to allow GUI components to be interrogated with gco / gcbo / findobj calls, but as I do not have control over components at creation, I cannot see how this might be achieved with a treetable. Any advice would be greatly appreciated
Kind regards
Thomas
#74 Comment By Yair Altman On December 21, 2016 @ 10:24
@Thomas – refer to the “Data Model” section in my post. You can set the Tag property of the hContainer handle that is returned by the javacomponent function call. The model itself can be retrieved and interrogated at run-time via
jTable.getModel
.If you want to learn more about how to work with javacomponent and/or interrogate the model, then read other posts on this blog, or read my [31], or get my [32].
If you need additional assistance for your specific project, contact me offline (altmany at gmail) for a short consultancy.
#75 Comment By Thomas On December 22, 2016 @ 11:43
Thanks for your response Yair. Playing with Java classes is a bit new to me but I will have a go.
All the best
Thomas
#76 Comment By Daniele F. On December 23, 2016 @ 12:23
Dear Yair,
First of all, thanks for this great tool.
Second: I’m not a Java user
Question:
At the end of your code you written some sample functions.
I wanted to use the %% Sample row deletion function.
So I tried the command:
but what I get is:
More than this, if I look into:
[34]
I don’t find any reference to the method removeRow
Is the removeRow function obsolete or am I, most probably, doing something wrong?
Kind regards
Daniele
#77 Comment By Yair Altman On December 23, 2016 @ 12:52
@Daniele – that code snippet is not connected to the main program, it was just illustrative (as in pseudo-code).
SortableTreeTableModel
does not have a removeRow method, but the underlying data model (aTreeTableModel
object, accessible viajtable.getModel.getActualModel
) does.#78 Comment By Daniele F. On February 14, 2017 @ 17:18
Dear Yair
Thanks for your answer. OK, so I tried the function with the wrong data model.
As far as I’ve understood, the java object created with the treeTable function is of class GroupTable.
Now on [35] it seems to me that the method setValueAt should be inherited from theclass javax.swing.JTable, but I’m not able to change the values on the table with this method.
E.g. for the command “handles.jtable.setValueAt(2)” the result is:
No method ‘setValueAt’ with matching signature found for class ‘com.jidesoft.grid.GroupTable’.
So now if I want to modify the values on the table, I’m modifying a saved *.mat file and I upload it for every modification, that seems to me not very efficient.
Can I ask you what am I missing or how can I directly modify the jtable?
Many thanks in advance
Daniele
#79 Comment By Yair Altman On February 14, 2017 @ 19:31
jTable.setValueAt() expects 3 input parameters, not 1.
General note: This is not a blog about Java or JTable, but on Matlab. You really need to search for answers about JTable and such in Java-related sites online – there are plenty of those around, Google would have given you the answer in less time than it took you to write your comment…
#80 Comment By Daniele F. On February 21, 2017 @ 12:15
Dear Yair
You’re completely right.
Thanks for having taken the time to answer to such a stupid question 🙂
#81 Comment By Shi On March 6, 2017 @ 08:36
Hi Yair
How to add button and callback in uitable and createtreetable.m
thanks
#82 Comment By ss On May 2, 2017 @ 06:57
Thanks ,I have no way to update the treetable ,when I use and jtable.setModel(javax.swing.table.DefaultTableModel(jData,jHeader));jtable.repaint; it can be shown in a treegroup ,but the other method can’t update the data,
and when I use jtable.getModel.getActualModel to addrow ,it can only add one string rather then we needed column number,insert also exist the trouble
and how to create a uicontextmenu to treetable ,I can’t find JPoPupmenu from the jtable
#83 Comment By ss On May 24, 2017 @ 04:08
Hi,Yair
I have a question how to add a column button to the treetable
#84 Comment By Daniele F. On November 16, 2017 @ 17:12
Dear Yair
I’m happily using your treeTable utility, thanks again to making it available.
I’ve a question:
I’ve compiled an application that uses the treeTable function. When I start the compiled application I receive the message:
“Unauthorized usage of JIDE products
You get this message box is because you didn’t input a correct license key. …”
Where do I have to insert the licence key to avoid this message? And which is the minimum purchase that I should buy in order to use the treeTable utility, if I have to? ( [36])
Thanks in advance
#85 Comment By Yair Altman On November 16, 2017 @ 17:31
@Daniele – I will answer you in an offline email
#86 Comment By paul On June 8, 2018 @ 00:05
Hi Yair,
This is great GUI table control. Just one question, I try to disable the header, what should I do? Or where can I find relative information about that?
Thanks for your help
Regards,
Paul
#87 Comment By Yair Altman On June 18, 2018 @ 02:38
@Paul – To hide the header row altogether:
To disable (but still show) the header:
#88 Comment By Alex On August 6, 2020 @ 14:17
Hi,
I was wondering if there is a possibility to integrate a text field with label and another table in one cell of the tree table, or is it restricted to ComboBoxes and Checkboxes? Is it possible to integrate Matlab’s uicontol( ) into the cells?
BR,
Alex
#89 Comment By Donato Coppola On November 22, 2020 @ 20:49
Hi Yair,
I have a problem with the double format.
When I run the treeTable function, the numbers in double format cells are displayed with comma as decimal separator.
How can I change it in a dot?
Thanks
Donato
#90 Comment By Donato Coppola On September 23, 2023 @ 12:14
Hi Yair!
I have a problem with the treetable.
I disabled the editing of the table, but if Itry to compile the app, this property is ignored and the table became editable. Is there a way to resolve the problem?
Thanks