We often need to display numbers, in GUI, text files or maybe the console window (Matlab Desktop). Matlab has built-in support for this using the fully-documented num2str, sprintf and format functions. Unfortunately, these built-in functions, useful as they are for simple needs, have several limitations:
- They do not take into account the user’s computer Locale settings. For example, the number 1234.56 is normally displayed as 1,234.56 or 1’234.56 or 1234,56 depending on your chosen Locale (which is determined by the local language and country). The functions always use the same manner to display the number, disregarding the user’s Locale.
- format does not enable customization of the number of decimal digits, and num2str and sprintf‘s ability to do so is limited. Customization of the decimal sign and thousands grouping sign is even more difficult.
I have recently completed a consulting work for a bank in Switzerland where these limitations were very important. The bank has branches in several countries and people naturally use different Locales on their computer. Moreover, some data values (for example, FOREX exchange rates) need more than two decimal digits. I had to find a generic cross-platform way to code this in Matlab in a way that will work for all users out-of-the-box (the application is compiled and distributed as an executable).
The solution was to use Matlab’s fully-documented built-in support for using Java objects. In this case, we shall use the standard java.text.DecimalFormat:
>> % Simple formatting example >> nf = java.text.DecimalFormat >> nf.format(1234567.890123) ans = 1,234,567.89 >> % Modify some properties... >> set(nf,'GroupingSize',5) >> nf.setMaximumFractionDigits(4) >> nf.format(1234567.890123) ans = 12,34567.8901
In the simple code snippet above, note the two alternative manners in which I set the DecimalFormat
object’s properties, once with the set(‘XYZ’,value) function, then using the corresponding Java accessor method setXYZ(value). In most cases, we find that Java properties can be set using either of these manners, although using the Java accessor methods is typically safer and prevents some possible problems. Similarly, property values can be retrieved using either get(‘XYZ’) or the corresponding getXYZ() or isXYZ() Java methods (which are better).
Some of the interesting gettable/settable properties of the DecimalFormat
object include (my Locale’s defaults are specified – your Locale might be different):
- Currency – a java.util.Currency object that sets the currency symbol, international currency code and number of fractional decimal digits
- DecimalFormatSymbols – a java.text.DecimalFormatSymbols object that sets the character symbols for such things as the decimal sign (“.” or “,” for example), grouping separator (“,” or ” ‘ ” or whatever), percent and per-mill signs, minus sign, infinity sign etc.
- DecimalSeparatorAlwaysShown – (default=false) a boolean flag that specifies whether or not to display the decimal sign even for integer numbers. For example, “123.” rather than “123”.
- GroupingSize – (default=3) indicates how many integer digits should be grouped by the grouping (“thousands”) separator sign.
- GroupingUsed – (default=true) a boolean flag that specifies whether or not to use the grouping sign at all. Matlab’s built-in num2str does not use grouping.
- MaximumFractionDigits – (default=3) the maximal number of digits to display to the right of the decimal sign. Post-rounding trailing zeros are omitted.
- MinimumFractionDigits – (default=0) the minimum number of digits to display, padding with trailing zeros if necessary.
- MaximumIntegerDigits – (default=intmax) the maximal number of digits to display to the left of the decimal sign. Extra digits are removed.
- MinimumIntegerDigits – (default=1) the minimum number of digits to display, padding with leading zeros if necessary.
- Multiplier – (default=1) multiplies the number by the specified value before converting to string.
- NegativePrefix – (default=’-‘) the string to display to the left of a negative number.
- NegativeSuffix – default = ”) the string to display to the right of a negative number. Some bankers like to display negative numbers as “(value)” and this is easy to do using NegativePrefix and NegativeSuffix. There are corresponding properties PositivePrefix and PositiveSuffix.
- RoundingMode – (default=RoundingMode.HALF_EVEN) a java.math.RoundingMode object that sets the manner in which numbers are rounded to fit the required number of digits.
In addition, the DecimalFormat
object provides methods that enable setting/getting a pattern that directly specifies how numbers should be parsed. A description of the different pattern components can be found here. The pattern directly corresponds to the settable properties listed above, and is very similar to the custom numeric pattern that is settable in Microsoft Excel. For example:
>> % default format: >> nf.toPattern ans = #,##0.### >> str = char(nf.format(-pi)) str = -3.142 >> % non-default format: >> nf.setNegativePrefix('- (') >> nf.setNegativeSuffix(') !!!') >> % alternatively: nf.applyPattern('#,##0.###;- (#,##0.###) !!!') >> nf.toPattern ans = #,##0.###;- (#,##0.###) !!! >> str = nf.format(-pi).char str = - (3.142) !!! >> % Alternatively, we could use the pattern directly: >> str = char(java.text.DecimalFormat('#,##0.###;- (#,##0.###) !!!').format(-pi));
Note how we convert the java.lang.String
object returned by the format method into a Matlab string using the built-in char function.
To test how the numbers react to different Locales, we could specify a specific Locale to the DecimalFormatSymbols
object, as follows:
>> dfs = java.text.DecimalFormatSymbols(java.util.Locale('fr-CH')); % Swiss-French Locale >> dfs.setGroupingSeparator(''''); % use ' rather than , >> nf.setDecimalFormatSymbols(dfs) >> nf.format(123456.789012) ans = 123'456.789
Alternatively, change the computer’s current Locale (in Windows, this is done via the Control Panel’s Regional Settings dialog), open a new Matlab session and rerun the code using the default DecimalFormat
. Restarting Matlab is important, because the Locale is only read once by the Java engine (JVM), when it starts, and this happens when Matlab starts.
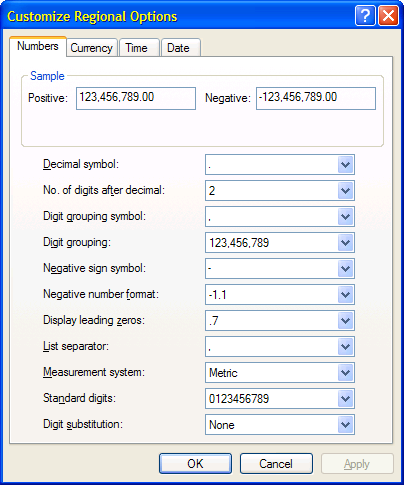
Window's Control Panel's Regional Settings Locale
To complete the picture, the DecimalFormat
object can not only format a numeric value as a string, using format() as we have seen above, but also the reverse – convert a string to a numeric value using parse():
>> value = nf.parse('123''456.789').doubleValue value = 123456.789
Do you have a solution to format doubles leaving n (say 4) significant digits for pos/neg numbers between for example [1e-5, 1e+5] and scientific notation for very large/small numbers like in example below?
0.000012345678 -> 1.235e-005
0.001234567898 -> 0.001235
0.123456789876 -> 0.1235
1.234567898765 -> 1.235
123.4567898765 -> 123.5
1234.567898765 -> 1235
123456.7898765 -> 123457
1234567.898765 -> 1.234e+006
except for your next to last example you could use the sprintf option as follows:
perhaps there are other solutions.
Donn
Pingback: Grouping Digits | Now
Is there also a way to force a specific scientific notation power?
For example instead of Matlab displaying:
0.001e-3
Make it show 1.123e-6 ?
This is important sometimes when small numbers are part of a vector with larger values.
Thanks,
Ori
@Ori – you need to do it programmatically, there is no immediate solution for displaying a vector with different exponents.
I need to format numbers with engineeering prefixes 1e3 to 1k, sometimes with units (1 kg).
Currently,I use DecimalFormat with the right prefix to get the exponent into groups of 3, than native matlab string handling and a hashtable to extract the correct prefic (exponent ‘3’ = ‘k’), all well and good. The FEX submission, num2sip (http://www.mathworks.com/matlabcentral/fileexchange/33174-number-to-scientific-prefix) does the same thing cleverer and all in native matlab.
My question is the use of a AttributedCharacterIterator and the attribute returned. The attribute returned is a java.text.NumberFormat.Field object but takes the form java.text.NumberFormat$Field(exponent field) and I can not seem to create a java.text.NumberFormat.Field object in matlab.
Any help would be appreciated
@Collin –