Continuing last week’s article on customized combo-boxes (a.k.a. popup menus or drop-downs), today I discuss how we can use JIDE‘s vast array of custom professional combo-boxes in our Matlab GUI.
As I’ve already noted here in several articles, Matlab heavily uses JIDE’s library of GUI controls for its own GUI (Desktop, Editor etc.) and we can tap into this wealth of components in our own GUI. I’ve recently shown an example of this in my treeTable utility, which greatly extends Matlab’s standard uitable. After using treeTable for a while, it’s difficult to go back to using the plain standard uitable… A similar experience will undoubtedly occur after using some of JIDE’s combo-boxes.
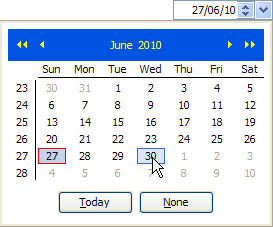
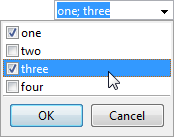
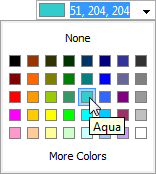
Some of the JIDE combo-box controls
JIDE combo-boxes
JIDE’s combo-box controls are generally grouped in the JIDE Grids package and extend the standard Java Swing JComponent
(JIDESoft actually found it easier to extend JComponent
than JComboBox
, but users really have nothing to complain – all the extra functionality and more have simply been reimplemented). The JIDE Grids package is included in each Matlab installation (/java/jarext/jide/jide-grids.jar under the Matlab root). In particular, JIDE Grids includes the com.jidesoft.combobox
Java package, which groups the combo-box components. You can find further details on JIDE Grids in the Developer Guide and the Javadoc documentation.
Back in 2010, I explained how we can use one of these components, DateComboBox
, and its close associate DateSpinnerComboBox
. Today I extend the coverage to briefly examine JIDE’s other custom combo-box controls.
Each of the following classes has both a combo-box control and a corresponding panel that is presented when the combo-box arrow button is clicked (activated). The panel is normally called xxxChooserPanel
and its corresponding combo-box is called xxxComboBox
. So, for example, we would have ColorComboBox
coupled with ColorChooserPanel
. After the combo-box is created, the panel can be accessed and customized via the combo-box’s PopupPanel property or the corresponding getPopupPanel() method. The panel can also be displayed as a standalone control, as I’ve shown in the DateComboBox
article. GUI designers can choose whether to use a compact combo-box or the full-size panel control.
ListComboBox
– a simple combo-box that accepts a list (cell array) of selectable values (e.g., string values). This is the simplest JIDE combo-box. It doesn’t add much over Matlab’s standard combo-box control except the ability to easily set the MaximumRowCount property (default value =8). On the other hand, using findjobj we can do the same thing with Matlab’s control…MultilineStringComboBox
– a combo box that enables entering a string item that has multiple lines of text. A similar control isStringArrayComboBox
that simply formats the multi-line string differently in the result, separating the multi-lines with a ‘;’ character.MultiSelectListComboBox
– a combo-box that enables selecting multiple items using a combination of <Shift>-click and <Ctrl>-clickMultiSelectListComboBox
CheckBoxListComboBox
– a combo-box that presents a multi-selection panel using check-boxes. It will be discussed in next week’s article.CheckBoxListComboBox
CalculatorComboBox
– a combo-box control that displays the result of simple arithmetic calculations. Possible customizations include the ability to set the calculator buttons’ size and gap, the displayed number format, the initial value etc. – all via the Calculator property that returns a customizablecom.jidesoft.swing.Calculator
object.CalculatorComboBox
ColorComboBox
– a combo-box control that enables color selection. Matlab includes a large number of color selectors, as explained in this article. Possible customizations of theColorComboBox
control include the ability to hide the color’s RGB value (ColorValueVisible property, boolean, default=true), hide the colored icon rectangle (ColorIconVisible property, boolean, default=true), make the control look like an editbox rather than a combo-box (ButtonVisible property, boolean, default=true), allow the “More colors…” features (AllowMoreColors, boolean, default=true) etc.ColorComboBox
JideColorSplitButton
– a control very similar toColorComboBox
, meant for usage in toolbars or wherever a narrow color-selection control is needed:JideColorSplitButton
DateComboBox
– presents a date-selection combo-box, whose attributes (day/month names, display format etc.) are taken from the system definitions. JIDE’s date-selection components were discussed in a dedicated article. Possible customizations include the ability to display an integrated time-selector sub-component (via the TimeDisplayed property: boolean, default=false and TimeFormat string), week numbers (ShowWeekNumbers, boolean, default=true), <OK> button (ShowOKButton, boolean, default=false), the <Today> button (ShowTodayButton, boolean, default=true) and the <None> button (ShowNoneButton, boolean, default=true).DateComboBox
DateSpinnerComboBox
– presents a date-selection combo-box that includes both theDateComboBox
and a spinner controlDateSpinnerComboBox
MonthComboBox
– a month-selection combo-box, similar toDateComboBox
but without the ability to select individual days, only full monthsMonthComboBox
FileChooserComboBox
– a combo-box that displays a file-selection window when activated. Customizations include the ability to set the CurrentDirectoryPath used by the popup window.FolderChooserComboBox
– a combo-box that displays a folder-selection window when activatedFontComboBox
– a font selection combobox (Matlab has several other font-selection components that we can use, in addition to uisetfont)FontComboBox
InsetsComboBox
– enables interactive definition of insets (margins)InsetsComboBox
TreeComboBox
– a combo-box that displays selectable data in tree format (i.e., a hierarchy of items)TreeComboBox
TableComboBox
– a combo-box that displays selectable data in table format (i.e., multiple columns). The combo-box’s value is the selected table row’s left-most column value. Here’s an example:data = num2cell([magic(3); -magic(3)]); % needs to be a 2D cell-array headers = {'one', 'two', 'three'}; tableModel = javax.swing.table.DefaultTableModel(data, headers); jCombo = com.jidesoft.combobox.TableComboBox(tableModel); [hjCombo, hContainer] = javacomponent(jCombo, [150,300,120,20], gcf);
TableComboBox
Integrating in Matlab GUI
To use any of these controls, we first need to initialize JIDE usage in Matlab, then create an instance of the control, and finally place it onscreen using the semi-documented javacomponent function:
% Initialize JIDE's usage within Matlab com.mathworks.mwswing.MJUtilities.initJIDE; % Display a DateChooserPanel jCombo = javaObjectEDT(com.jidesoft.combobox.DateComboBox); % javaObjectEDT is optional but advisable [hjCombo, hContainer] = javacomponent(jCombo, [10,10,100,20], gcf)
Each combo-box control accepts its combo-list elements somewhat differently. For example, for DateComboBox
we can specify the acceptable date-range (via DefaultDateModel
) and initial date, for a FontComboBox
we can specify the initial font, and for any of the list controls we need to specify the list elements (as a cell-array of strings). However, most if not all of these components have default constructors so we can initialize the controls without any inputs and let it automatically use the defaults. For example, the date-selection controls will use the current date (today) as its default value.
In most cases, we can set the control handle’s ActionPerformedCallback to process a selection event (or modification of the combo-box value via direct editing in its integrated editbox):
set(hjCombo, 'ActionPerformedCallback', @myMatlabCallbackFcn);
The selected item can in most cases be retrieved via
selectedItem = get(hjCombo,'SelectedItem'); % or: hjCombo.getselectedItem
For some combo-boxes, non-numeric results may need to be converted into Matlab strings, via the char function:
selectedItem = char(selectedItem);
Next week I plan to present a non-trivial customization of the CheckBoxListComboBox
control.
Additional discussion of JIDE’s combo-boxes, and JIDE controls in general, is available in Chapter 5 of my Matlab-Java Programming book.
If you need to integrate professional-looking controls such as these in your Matlab GUI, consider hiring my consulting services.
Caution
Remember that JIDE evolves with Matlab, and so JIDE’s online documentation, which refers to the latest JIDE version, may be partially inapplicable if you use an old Matlab version. In any case, Matlab releases always lag the latest JIDE release by at least a year (e.g., Matlab R2013b uses JIDE v3.4.1 that was released in June 2012). The older your Matlab, the more such inconsistencies that you may find. For example, I believe that DateSpinnerComboBox
only became available around R2010b; similarly, some control properties behave differently (or are missing altogether) in different releases. To determine the version of JIDE that you are currently using in Matlab, run the following (the result can then be compared to JIDE’s official change-log history):
>> com.jidesoft.utils.Lm.getProductVersion ans = 3.4.1
Note that JIDE is a commercial product. We may not use it without JIDESoft’s permission outside the Matlab environment. It is my understanding however, that we can freely use it within Matlab. Note that this is not legal advise as I am an engineer, not a lawyer. If you have any licensing questions, contact sales@jidesoft.com.
Hi Yair,
I was trying to use a â—¾TreeComboBox in my GUI, but it seems something is wrong:
hi…
1)how can i set string to CheckBoxListComboBox and get index of selected string
2)is other kind calender of DateComboBox related to jalili calender