Last month, I posted an article that summarized a variety of undocumented customizations to Matlab figure windows. As I noted in that post, Matlab figures have used Java JFrame
s as their underlying technology since R14 (over a decade ago), but this is expected to change a few years from now with the advent of web-based uifigures. uifigures first became available in late 2014 with the new App Designer preview (the much-awaited GUIDE replacement), and were officially released in R2016a. AppDesigner is actively being developed and we should expect to see exciting new features in upcoming Matlab releases.
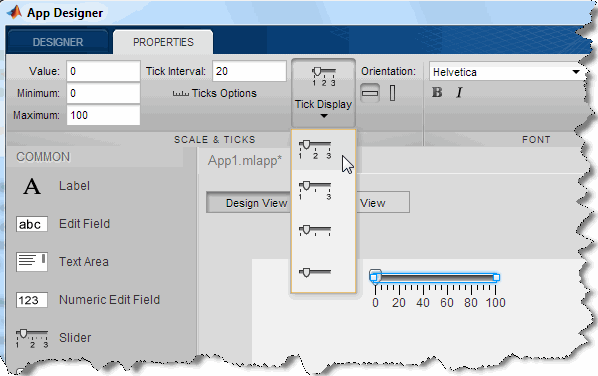
Matlab's new AppDesigner (a somewhat outdated screenshot)
However, while AppDesigner has become officially supported, the underlying technology used for the new uifigures remained undocumented. This is not surprising: MathWorks did a good job of retaining backward compatibility with the existing figure handle, and so a new uifigure returns a handle that programmatically appears similar to figure handles, reducing the migration cost when MathWorks decides (presumably around 2018-2020) that web-based (rather than Java-based) figures should become the default figure type. By keeping the underlying figure technology undocumented and retaining the documented top-level behavior (properties and methods of the figure handle), Matlab users who only use the documented interface should expect a relatively smooth transition at that time.
So does this mean that users who start using AppDesigner today (and especially in a few years when web figures become the default) can no longer enjoy the benefits of figure-based customization offered to the existing Java-based figure users (which I listed in last month’s post)? Absolutely not! All we need is to get a hook into the uifigure‘s underlying object and then we can start having fun.
The uifigure Controller
One way to do this is to use the uifigure handle’s hidden (private) Controller property (a matlab.ui.internal.controller.FigureController
MCOS object whose source-code appears in %matlabroot%/toolbox/matlab/uitools/uicomponents/components/+matlab/+ui/+internal/+controller/).
Controller is not only a hidden but also a private property of the figure handle, so we cannot simply use the get function to get its value. This doesn’t stop us of course: We can get the controller object using either my getundoc utility or the builtin struct function (which returns private/protected properties as an undocumented feature):
>> hFig = uifigure('Name','Yair', ...); >> figProps = struct(hFig); % or getundoc(hFig) Warning: Calling STRUCT on an object prevents the object from hiding its implementation details and should thus be avoided. Use DISP or DISPLAY to see the visible public details of an object. See 'help struct' for more information. (Type "warning off MATLAB:structOnObject" to suppress this warning.) Warning: figure JavaFrame property will be obsoleted in a future release. For more information see the JavaFrame resource on the MathWorks web site. (Type "warning off MATLAB:HandleGraphics:ObsoletedProperty:JavaFrame" to suppress this warning.) figProps = JavaFrame: [] JavaFrame_I: [] Position: [87 40 584 465] PositionMode: 'auto' ... Controller: [1x1 matlab.ui.internal.controller.FigureController] ControllerMode: 'auto' ... >> figProps.Controller ans = FigureController with properties: Canvas: [] ProxyView: [1x1 struct] >> figProps.Controller.ProxyView ans = PeerNode: [1x1 com.mathworks.peermodel.impl.PeerNodeImpl] PeerModelManager: [1x1 com.mathworks.peermodel.impl.PeerModelManagerImpl] >> struct(figProps.Controller) Warning: Calling STRUCT on an object prevents the object from hiding its implementation details and should thus be avoided. Use DISP or DISPLAY to see the visible public details of an object. See 'help struct' for more information. (Type "warning off MATLAB:structOnObject" to suppress this warning.) ans = PositionListener: [1x1 event.listener] ContainerPositionCorrection: [1 1 0 0] Container: [1x1 matlab.ui.internal.controller.FigureContainer] Canvas: [] IsClientReady: 1 PeerEventListener: [1x1 handle.listener] ProxyView: [1x1 struct] Model: [1x1 Figure] ParentController: [0x0 handle] PropertyManagementService: [1x1 matlab.ui.internal.componentframework.services.core.propertymanagement.PropertyManagementService] IdentificationService: [1x1 matlab.ui.internal.componentframework.services.core.identification.WebIdentificationService] EventHandlingService: [1x1 matlab.ui.internal.componentframework.services.core.eventhandling.WebEventHandlingService]
I will discuss all the goodies here in a future post (if you are curious then feel free to start drilling in there yourself, I promise it won’t bite you…). However, today I wish to concentrate on more immediate benefits from a different venue:
The uifigure webwindow
uifigures are basically webpages rather than desktop windows (JFrames). They use an entirely different UI mechanism, based on HTML webpages served from a localhost webserver that runs CEF (Chromium Embedded Framework version 3.2272 on Chromium 41 in R2016a). This runs the so-called CEF client (apparently an adaptation of the CefClient sample application that comes with CEF; the relevant Matlab source-code is in %matlabroot%/toolbox/matlab/cefclient/). It uses the DOJO Javascript toolkit for UI controls visualization and interaction, rather than Java Swing as in the existing JFrame figures. I still don’t know if there is a way to combine the seemingly disparate sets of GUIs (namely adding Java-based controls to web-based figures or vice-versa).
Anyway, the important thing to note for my purposes today is that when a new uifigure is created, the above-mentioned Controller object is created, which in turn creates a new matlab.internal.webwindow
. The webwindow class (%matlabroot%/toolbox/matlab/cefclient/+matlab/+internal/webwindow.m) is well-documented and easy to follow (although the non camel-cased class name escaped someone’s attention), and allows access to several important figure-level customizations.
The figure’s webwindow
reference can be accessed via the Controller‘s Container‘s CEF property:
>> hFig = uifigure('Name','Yair', ...); >> warning off MATLAB:structOnObject % suppress warning (yes, we know it's naughty...) >> figProps = struct(hFig); >> controller = figProps.Controller; % Controller is a private hidden property of Figure >> controllerProps = struct(controller); >> container = controllerProps.Container % Container is a private hidden property of FigureController container = FigureContainer with properties: FigurePeerNode: [1x1 com.mathworks.peermodel.impl.PeerNodeImpl] Resizable: 1 Position: [86 39 584 465] Tag: '' Title: 'Yair' Icon: 'C:\Program Files\Matlab\R2016a\toolbox\matlab\uitools\uicomponents\resources\images…' Visible: 1 URL: 'http://localhost:31417/toolbox/matlab/uitools/uifigureappjs/componentContainer.html…' HTML: 'toolbox/matlab/uitools/uifigureappjs/componentContainer.html' ConnectorPort: 31417 DebugPort: 0 IsWindowValid: 1 >> win = container.CEF % CEF is a regular (public) hidden property of FigureContainer win = webwindow with properties: URL: 'http://localhost:31417/toolbox/matlab/uitools/uifigureappjs/component…' Title: 'Yair' Icon: 'C:\Program Files\Matlab\R2016a\toolbox\matlab\uitools\uicomponents\re…' Position: [86 39 584 465] CustomWindowClosingCallback: @(o,e)this.Model.hgclose() CustomWindowResizingCallback: @(event,data)resizeRequest(this,event,data) WindowResizing: [] WindowResized: [] FocusGained: [] FocusLost: [] DownloadCallback: [] PageLoadFinishedCallback: [] MATLABClosingCallback: [] MATLABWindowExitedCallback: [] PopUpWindowCallback: [] RemoteDebuggingPort: 0 CEFVersion: '3.2272.2072' ChromiumVersion: '41.0.2272.76' isWindowValid: 1 isDownloadingFile: 0 isModal: 0 isWindowActive: 1 isAlwaysOnTop: 0 isAllActive: 1 isResizable: 1 MaxSize: [] MinSize: [] >> win.URL ans = http://localhost:31417/toolbox/matlab/uitools/uifigureappjs/componentContainer.html?channel=/uicontainer/393ed66a-5e34-41f3-8ac0-0b0f3b0738cd&snc=5C2353
An alternative way to get the webwindow
is via the list of all webwindow
s stored by a central webwindowmanager
:
webWindows = matlab.internal.webwindowmanager.instance.findAllWebwindows(); % manager method returning an array of all open webwindows webWindows = matlab.internal.webwindowmanager.instance.windowList; % equivalent alternative via manager's windowList property
Note that the controller, container and webwindow class objects, like most Matlab MCOS objects, have internal (hidden) properties/methods that you can explore. For example:
>> getundoc(win) ans = Channel: [1x1 asyncio.Channel] CustomEventListener: [1x1 event.listener] InitialPosition: [100 100 600 400] JavaScriptReturnStatus: [] JavaScriptReturnValue: [] NewWindowBeingCreated: 0 NewWindowCreated: 1 UpdatedPosition: [86 39 584 465] WindowHandle: 2559756 newURL: 'http://localhost:31417/toolbox/matlab/uitools/uifigureappjs/componentContai…'
Using webwindow for figure-level customizations
We can use the methods of this webwindow
object as follows:
win.setAlwaysOnTop(true); % always on top of other figure windows (a.k.a. AOT) win.hide(); win.show(); win.bringToFront(); win.minimize(); win.maximize(); win.restore(); win.setMaxSize([400,600]); % enables resizing up to this size but not larger (default=[]) win.setMinSize([200,300]); % enables resizing down to this size but not smaller (default=[]) win.setResizable(false); win.setWindowAsModal(true); win.setActivateCurrentWindow(false); % disable interaction with this entire window win.setActivateAllWindows(false); % disable interaction with *ALL* uifigure (but not Java-based) windows result = win.executeJS(jsStr, timeout); % run JavaScript
In addition to these methods, we can set callback functions to various callbacks exposed by the webwindow
as regular properties (too bad that some of their names [like the class name itself] don’t follow Matlab’s standard naming convention, in this case by appending “Fcn” or “Callback”):
win.FocusGained = @someCallbackFunc; win.FocusLost = @anotherCallbackFunc;
In summary, while the possible customizations to Java-based figure windows are more extensive, the webwindow
methods appear to cover most of the important ones. Since these functionalities (maximize/minimize, AOT, disable etc.) are now common to both the Java and web-based figures, I really hope that MathWorks will create fully-documented figure properties/methods for them. Now that there is no longer any question whether these features will be supported by the future technology, and since there is no question as to their usefulness, there is really no reason not to officially support them in both figure types. If you feel the same as I do, please let MathWorks know about this – if enough people request this, MathWorks will be more likely to add these features to one of the upcoming Matlab releases.
Warning: the internal implementation is subject to change across releases, so be careful to make your code cross-release compatible whenever you rely on one of Matlab’s internal objects.
Note that I labeled this post as “part 1” – I expect to post additional articles on uifigure customizations in upcoming years.
Thanks a lot for your posts!
I’m currently beginning to create my first really big Matlab GUI and I still dont know, if I should use the new App Designer. The new App Designer has some very cool features, however, it also offers not the full functionality of the old programmatically way. For instance, no menu bar is available in App Designer, which is really annoying…
Maybe you could share your opinion on the pros and cons of App Designer in future posts, or maybe you find even an undocumented menu bar 😉
Anyway, thumbs up for your blog!
Ben
@Ben – at the moment, using programmatic GUI creation that relies on the old Java frame enables far greater customizability and functionality than the new web-based uifigures created by AppDesigner (AD). AD does offer an easy-to-use GUI layout editor and a few nice-looking widgets (knobs, dials and switches), but it also [currently] lacks many important functionalities compared to the old figures and controls. Moreover, if you know how to programmatically create and modify Matlab GUIs then you don’t need AD’s widgets, and if you know how to integrate Java widgets in Matlab GUIs then you could use one of the zillion widgets available online. So in essence, AppDesigner is excellent for simple GUI development, but not [currently] for highly-polished professional-looking GUIs.
That being said, AppDesigner is constantly being improved with each Matlab release. I’m almost certain that menus and toolbars will be added to uifigures soon. At some point in the near future I expect AD to be at least as powerful and useful as programmatic
JFrame
-based GUIs. There would always be some stuff that we would never be able to do with uifigures, but the time will soon come when AD and uifigure‘s benefits will outweigh those limitations. My guesstimate is that we’re ~1 year away from that point for simple GUIs, and ~2 years away for most advanced GUIs.Of course, the importance of various features is different for different users, so for some users the balance point may have already arrived, and for others it may never arrive…
Thanks a lot for your thoughts!
If anybody is interested in a comparison of App Designer vs Standard figures in 2016a, I recommend these links here:
https://de.mathworks.com/matlabcentral/answers/279042-app-designer-s-editor-is-slow-and-gets-stuck-alot?s_tid=srchtitle
https://de.mathworks.com/matlabcentral/answers/283215-insert-image-in-appdesigner-gui?s_tid=srchtitle
https://de.mathworks.com/matlabcentral/answers/289578-how-to-share-data-between-gui-from-guide-and-app-from-app-designer?s_tid=srchtitle
https://de.mathworks.com/matlabcentral/answers/275827-how-to-zoom-in-out-on-the-figures-in-app-designer?s_tid=srchtitle
Ben
Update: if you are running MATLAB 2017a, you can reach the FCE properties in this way:
Enjoy hacking AppDesigner 😉
Dear Davide Miani,
According to your update, I can change the ico to my own ico file by:
But I find it did not work when I added the following code to function startupFcn(app)
Hl Shi,
by installing the 1 sec pause, I was able to get the controller array loaded. Otherwise it is empty. Still poking around with it however it did work. just set MyICO to your ‘Filename.ico’ file. Note: must use correct file format. Java would let you use a png but this is an easy conversion.
Hi everyone, I’m having trouble creating a mlapp that has a popup that is a mlapp also.
So in other others I have 2 appdesigner apps… “main” and “popup”.
when I press a button on the main I want the popup to well.. popup.. and act as a modal window…
so I cant continue using the main app until I close the popup one.
I tried messing around with the:
win.setWindowAsModal(true);
with no luck… can someone give me some tips? 😉