8 years ago I blogged about Matlab’s builtin HelpPopup widget. This control is used by Matlab to display popup-windows with help documentation, but can also be used by users to display custom lightweight popups that contain HTML-capable text and even URLs of entire webpages. Today I’d like to highlight another builtin Matlab widget, ctrluis.PopupPanel
, which can be used to display rich contents in a lightweight popup box attached to a specific Matlab figure:
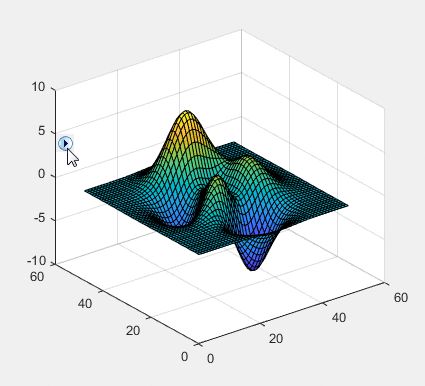
As you can see, this popup-panel displays richly-formatted contents, having either an opaque or transparent background, with vertical scrollbars being applied automatically. The popup pane is not limited to displaying text messages – in fact, it can display any Java GUI container (e.g. a settings panel). This popup-panel is similar in concept to the HelpPopup widget, and yet much more powerful in several aspects.
Creating the popup panel
Creating a PopupPanel
is very simple:
% Create the popup-panel in the specified figure hPopupPanel = ctrluis.PopupPanel(gcf); % use gcf or any figure handle hPopupPanel.setPosition([.1,.1,.8,.8]); % set panel position (normalized units) % Alternative #1: set popup-panel's contents to some HTML-formatted message % note: createMessageTextPane() has optional input args FontName (arg #2), FontSize (#3) jPanel = ctrluis.PopupPanel.createMessageTextPane('testing <b><i>123</i></b> ...') hPopupPanel.setPanel(jPanel); % Alternative #2: set popup-panel's contents to a webpage URL url = 'http://undocumentedmatlab.com/files/sample-webpage.html'; jPanel = javaObjectEDT(javax.swing.JEditorPane(url)); hPopupPanel.setPanel(jPanel); |
The entire contents are embedded within a scroll-box (which is a com.mathworks.widgets.LightScrollPane
object) whose scrollbars automatically appear as-needed, so we don’t need to worry about the contents fitting the allocated space.
To display custom GUI controls in the popup, we can simply contain those GUI controls in a Java container (e.g., a JPanel
) and then do hPopupPanel.setPanel(jPanel)
. This functionality can be used to create unobtrusive settings panels, input dialogs etc.
The nice thing about the popup widget is that it is attached to the figure, and yet is not assigned a heavyweight window (so it does not appear in the OS task-bar). The popup moves along with the figure when the figure is moved, and is automatically disposed when the figure is closed.
A few caveats about the ctrluis.PopupPanel
control:
- The widget’s parent is expected to be a figure that has pixel units. If it doesn’t, the internal computations of
ctrluis.PopupPanel
croak. - The widget’s position is specified in normalized units (default: [0,0,1,1]). This normalized position is only used during widget creation: after creation, if you resize the figure the popup-panel’s position remains unchanged. To modify/update the position of the popup-panel programmatically, use
hPopupPanel.setPosition(newPosition)
. Alternatively, update the control’s Position property and then callhPopupPanel.layout()
(there is no need to calllayout
when you usesetPosition
). - This functionality is only available for Java-based figures, not the new web-based (AppDesigner) uifigures.
Popup panel customizations
We can open/close the popup panel by clicking on its icon, as shown in the screenshots above, or programmatically using the control’s methods:
% Programmatically open/close the popup-panel hPopupPanel.showPanel; hPopupPanel.hidePanel; % Show/hide entire popup-panel widget (including its icon) hPopupPanel.setVisible(true); % or .setVisible(1) or .Visible=1 hPopupPanel.setVisible(false); % or .setVisible(0) or .Visible=0 |
To set a transparent background to the popup-panel (as shown in the screenshots above), we need to unset the opacity of the displayed panel and several of its direct parents:
% Set a transparent popup-panel background for idx = 1 : 6 jPanel.setOpaque(false); % true=opaque, false=transparent jPanel = jPanel.getParent; end jPanel.repaint |
Note that in the screenshots above, the panel’s background is made transparent, but the contained text and image remain opaque. Your displayed images can of course contain transparency and animation, if this is supported by the image format (for example, GIF).
iptui.internal.utilities.addMessagePane
ctrluis.PopupPanel
is used internally by iptui.internal.utilities.addMessagePane(hFig,message)
in order to display a minimizable single-line message panel at the top of a specified figure:
hPopupPanel = iptui.internal.utilities.addMessagePane(gcf, 'testing <b>123</b> ...'); % note the HTML formatting |
The function updates the message panel’s position whenever the figure’s size is modified (by trapping the figure’s SizeChangedFcn), to ensure that the panel is always attached to the top of the figure and spans the full figure width. This is a simple function so I encourage you to take a look at its code (%matlabroot%/toolbox/images/imuitools/+iptui/+internal/+utilities/addMessagePane.m) – note that this might require the Image Processing Toolbox (I’m not sure).
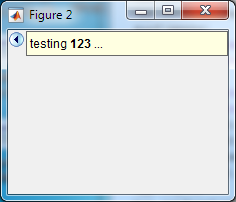
Professional assistance anyone?
As shown by this and many other posts on this site, a polished interface and functionality is often composed of small professional touches, many of which are not exposed in the official Matlab documentation for various reasons. So if you need top-quality professional appearance/functionality in your Matlab program, or maybe just a Matlab program that is dependable, robust and highly-performant, consider employing my consulting services.
I wonder if the popup panel (or a regular panel) can be used to display this type of embedded item
https://api.altmetric.com/embeds.html
I’ll include it here without the arrow marks marks because other wise the text doesn’t display. Instead of , I’ll use [].
[script type=’text/javascript’ src=’https://d1bxh8uas1mnw7.cloudfront.net/assets/embed.js’][/script]
[div class=’altmetric-embed’ data-badge-type=’donut’ data-doi=”10.1038/nature.2012.9872″][/div]
on a website displays the altmetric donut with the score associated with the doi address
I have tried to get matlab to display it without any luck.
I don’t know anything about java, which seems to be the problem.