A couple of weeks ago, a reader here asked how to integrate images in Matlab labels. I see quite a few queries about this, so I wanted to take today’s opportunity to explain how this can be done, and how to avoid common pitfalls.
In fact, there are two main methods of displaying images in Matlab GUI – the documented method, and the undocumented one:
The documented method
Some Matlab uicontrols (buttons, radio and checkboxes) have the CData property that can be used to load and display an image. For example:
imgData = imread('myImage.jpg'); % or: imread(URL) hButton = uicontrol('CData',imgData, ...); |
While label uicontrols (uicontrol(‘style’,’text’, …)) also have the CData property, it has no effect on these controls. Instead, we can create an invisible axes that displays the image using the image function.
The undocumented method
web-based images
I’ve already written extensively about Matlab’s built-in support for HTML in many of its controls. The supported HTML subset includes the <img> tag, and can therefore display images. For example:
htmlStr = '<html><b>Logo</b>: <img src="http://undocumentedmatlab.com/images/logo_68x60.png"/></html>'; hButton = uicontrol('Position',[10,10,120,70], 'String',htmlStr, 'Background','white'); |
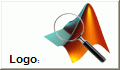
local images
Note that the image src (filename) needs to be formatted in a URL-compliant format such as 'http://www.website.com/folder/image.gif'
or 'file:/c:/folder/subfolder/img.png'
. If we try to use a non-URL-format filename, the image will not be rendered, only a placeholder box:
uicontrol('Position',..., 'String','<html><img src="img.gif"/></html>'); %bad uicontrol('Style','list', ... 'String',{...,'<html><img src="img.gif"/></html>'}); %bad |


Instead, we need to use correct URI syntax when specifying local images, which means using the
'file:/'
protocol prefix and the '/'
folder separator:
>> iconsFolder = fullfile(matlabroot,'/toolbox/matlab/icons/'); >> iconUrl = strrep(['file:/' iconsFolder 'matlabicon.gif'],'\','/'); >> str = ['<html><img src="' iconUrl '"/></html>'] str = <html><img src="file:/C:/Program Files/MATLAB/ ..... /icons/matlabicon.gif"/></html> >> uicontrol('Position',..., 'String',str); >> uicontrol('Style','list', ... str}); |


A similar pitfall exists when trying to integrate images in GUI control tooltips. I already discussed this issue here.
You can use HTML to resize the images, using the <img> tag’s width, height attributes. However, beware that enlarging an image might introduce pixelization effects. I discussed image resizing here – that article was in the context of menu-item icons, but the discussion of image resizing also applies in this case.
images in text labels
As for text labels, since text-style uicontrols do not unfortunately support HTML, we can use the equivalent Java JLabel
s, as I have explained here. Here too, we need to use the 'file:/'
protocol prefix and the '/'
folder separator if we want to use local files rather than internet files (http://…).
Java customizations
Using a uicontrol’s underlying Java component, we can customize the displayed image icon even further. For example, we can specify a different icon for selected/unselected/disabled/hovered/pressed/normal button states, as I have explained here. In fact, we can even specify a unique icon that will be used for the mouse cursor when it hovers on the control:


R2019b and newer
Iin R2019b and later, unless you specify the image size nothing will be shown. A solution is presented in this answer
str = ['<html><img src="file:/' iconUrl '" height="16" width="16"></html>']; |
Hi Dear Yair
That was nice point…
Thanks so much for your help.
Dear Yair,
First, sorry, my petition is not related to this entry, but I didn’t know where to post it.
I use error(‘…’) when I want to stop execution of a running m-file and show an error message. However, together with the error message, Matlab displays on screen all the other info with the line number or the mfile name where the error occurred (“Error in ==>..”). This distracts from the error message, and the end-user doesn’t care about all this info. I only want to display a message and stop execution. For example:
with pcoded files the extra info is not shown, but, in a non-compiled mfile, how to make "error(…)" not display all the extra info? Any suggestions are very welcome. Thank you very much.
@Peter – place a try-catch block in the main function of your application:
Thank you very much for your answer. Very appreciated.
Hi there Yair,
thanks for this very usefull post. But I have an ongoing question for this topic:
Is it possible to align text and image vertically centered?
Because in my Buttons, the text is aligned strictly at the bottom of the image. It would look more “professional” when I could align the text centered to the image.
@Stetsc – yes, we can do this using the underlying Java component:
This way, we can separately set the text and image locations in many different manners (left/center/right, top/center/bottom). I explain all these options in detail in section 6.1 of my Matlab-Java programming book.
Hi Yair,
thanks for the quick answer. I tried out, what you told me, but I can’t see any changes in the alignment of the text and image on the Button.
Here is my code:
I’m using MATLAB R2009b. Where is my fault?
Greetings,
stetsc
not:
Also, to use HTML formatting as you are obviously trying to do, you need to add “<html>” to the beginning of your string:
Oh, I just saw, that your comment-system interprets the HTML-Tags in my first codeline. And with this, you cannot see what I really coded. So I try it this way:
As you can see, I build the string out of the descriptive string “Beschreibung der gewählten Messgröße:” and the variable “img_path_info”.
So why it does not work?
I have to mention, that the Text and the image appear on the Button, side by side, as I want them to be, but the vertical positions are not really centered. The text is to low and the image to high.
Thanks for your help.
HTML has its own way of aligning the items, just as on a webpage. If you want to have more control then you need to set the image not via HTML but using the uicontrol’s CData property, and then you can control the placement of them separately as I have shown above.
Ah! I understand. Yair, you are my man!
Now it looks like I want it to. Many thanks for your help.
Here is the final code for all those who have the same problem out there:
And now the button has the image on the left, followed by the descriptive text. Both are vertically centered.
So again, thanks to you, Yair.
Greetings,
stetsc
I forgot one codeline:
Just needs to be added to the previous posted code.
Sorry 🙂
stetsc
instead of using
setIcon
you could simply use the CData property:Hi!
How can I save the cdata as a gif? I want to use the Icons outside Matlab.
Thx!
@Emanuel – use the built-in imwrite function
Hi Yair,
is it possible to display HTML text on axes ?
@Asaf – Matlab axes only support tex/latex. For example, to get bold font using Tex,
Additional information: http://www.mathworks.com/help/matlab/ref/text-properties.html
thank you 🙂
Dear Yair,
thank you for the detailed description. Do you see a chance to put a 90 deg rotated text on a uicontrol (pushbutton)?
Best regars
Klaus
@Klaus – not directly, but you can create a small image and add it to the button’s CData. As long as you don’t resize the button too much in either direction, this should work nicely.
Do you know a way to show images on push buttons via GUIDE?
Thanks in advance!
@Ronit – you can update the button’s CData property. Read the Matlab documentation for the uicontrol function for details/examples.
Hi Yair
I added image to my push button using the CData property but was not able to set its position.
The image added by me is positioned at the center of the button by default. Is there any way to change its position to bottom of the push button
@Sneha – either enlarge your image by adding empty space at the top, or use Java by accessing the button’s underlying Java control and then calling setVerticalAlignment(javax.swing.SwingConstants.BOTTOM).
Hi Yair,
Tried enlarging my image and added empty space on top of the image and this worked. Thank you for the help. I wanted to align my text and tried using the following lines
Can you please help me with this.
You need to use findjobj, not findobj. Read the post (and the other articles on this blog) carefully.
Hi, I am using MATLAB 2016 version
To anybody attempting this in R2019b (and later?): unless you specify the image size, nothing will be shown!. A solution is presented in this answer